Tkinter Scale – Command
Tkinter Scale command option is used to set a callback function for the value change event of the Scale widget. The command or callback function is called when the slider on the Scale widget is dragged.
We have to define this command or callback function before we can use it for the Scale command option.
Now, let us see how we can set this command option for the Scale widget.
We can set a command function while creating the Scale widget as shown below.
scale_1 = tk.Scale(window, command=on_scale_changed)
We have set the command option for the Scale widget with the on_scale_changed function. The on_scale_changed function has a single parameter to receive the value of the Scale as argument.
def on_scale_changed(value):
print("Scale value:", value)
Instead of the print statement, you may write your own business logic.
You can also set the command option with a function after creating the Scale widget as shown in the following.
scale_1 = tk.Scale(window)
scale_1['command'] = on_scale_changed
In this tutorial, you shall learn how to set the command option for a Scale widget in Tkinter, with examples.
Example
In this example, we shall create a Scale widget with command option set with the function on_scale_changed. Inside this function, we shall print the current value of the Scale.
Python Program
import tkinter as tk
# Callback function for Scale value change event
def on_scale_changed(value):
print(value)
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Create a Scale widget
scale_1 = tk.Scale(window, command=on_scale_changed)
scale_1.pack()
# Start the Tkinter event loop
window.mainloop()
CopyOutput in MacOS
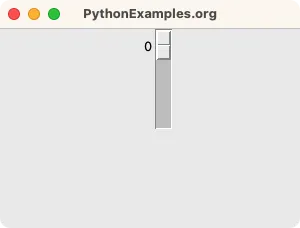
User slides the thumb on the Scale to set a new value.
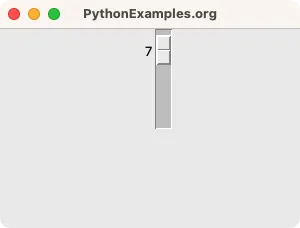
The command function on_scale_changed is called, and prints the value of the Scale to output.
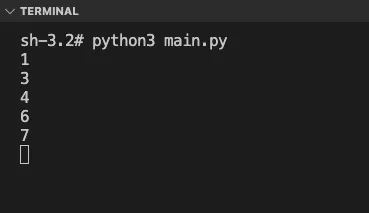
Summary
In this Python Tkinter tutorial, we have seen how to set command option for the Scale widget in Tkinter, with examples.