Tkinter Scale widget
In this tutorial, you shall learn about Scale widget in TKinter: What is a Scale widget, how to create a scale widget, and an example for scale widget.
What is Scale Widget in Tkinter
Scale widget is used to create a slider control that allows the user to select a value from a range. In a GUI, a Scale widget would be as shown in the following screenshot.
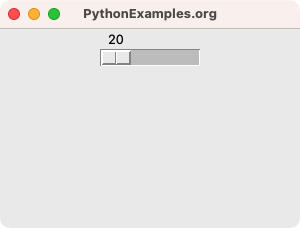
User can move the slider on the track and change the value of Scale.
Using the options of the scale widget, we can change the orientation, range of the values that we can select, styling, and other attributes of the Scale widget.
How to create a Scale widget in Tkinter?
To create a Scale widget in Tkinter, you can use the Scale class constructor. The parent window must be passed as the first argument, and this is the mandatory parameter. Rest of the parameters are optional and can be passed as keyword arguments, or you can set them after initializing the Scale widget object.
A simple example to create a Scale widget is given below.
import tkinter as tk
window = tk.Tk()
scale_1 = tk.Scale(window)
Now, let us specify some of the parameters.
scale_1 = tk.Scale(window, from_=0, to=100, orient=tk.HORIZONTAL, command=on_scale_changed)
- window specifies the parent for this Scale widget.
- from_ specifies the starting of the range.
- to specifies the ending of the range.
- orient specifies the orientation of the Scale widget: horizontal or vertical.
- command specifies the callback function for the value change event.
Example for Scale widget in Tkinter
In this example, we shall create a simple GUI application using Tkinter with a Scale widget.
Python Program
import tkinter as tk
# Callback function for Scale value change event
def on_scale_changed(value):
print(value)
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Create a Scale widget
scale_1 = tk.Scale(window, from_=20, to=40, orient=tk.HORIZONTAL, command=on_scale_changed)
scale_1.pack()
# Start the Tkinter event loop
window.mainloop()
As given in the previous section, we have created Scale widget scale_1 with Tk window as parent, set the from_ and to boundaries of the range of values that we can select with this scale, the orientation, and the command function.
Output in MacOS
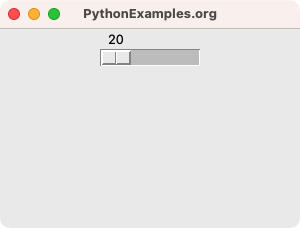
Summary
In this Python Tkinter tutorial, we have seen what a Scale widget is, how to create a Scale widget, and an example for Scale widget.