Contents
Tkinter simpledialog – Prompt for user input
In Tkinter, the simpledialog.askstring() method is used to prompt user for input, in a simple dialog box.
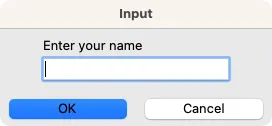
The user can enter a value in the entry field, and click on OK button. Then the entered value is returned by the simpledialog.askstring() method.
But, if the user clicks on Cancel, the simpledialog.askstring() method returns None.
In this tutorial, you will learn how to prompt user for an input in a simple dialog box using simpledialog.askstring() method in Tkinter, with an example program.
Syntax of simpledialog.askstring()
The syntax of askstring() method is
simpledialog.askstring(title, prompt)
where
Parameter | Description |
---|---|
title | This string is displayed as title of the dialog box. |
prompt | This string is displayed before the entry field. |
Examples
1. Read name of the user using Simple Dialog, and display greeting
Consider a simple use case where you would like to get the name of the user by prompting for input, read the input, and print a personalized greeting on the main window.
Python Program
import tkinter as tk
from tkinter import simpledialog
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
# Read input string from user
input_value = simpledialog.askstring("Input", "Enter your name")
print(input_value)
# Display entered value in a label
user_name = input_value if input_value is not None else "User"
label = tk.Label(window, text="Hello " + user_name + "!")
label.pack()
# Run the application
window.mainloop()
Output in Windows
Output in MacOS
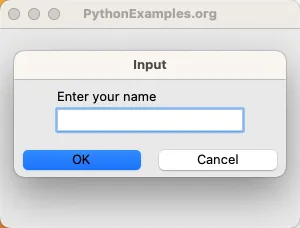
If user enters an input value in the field.
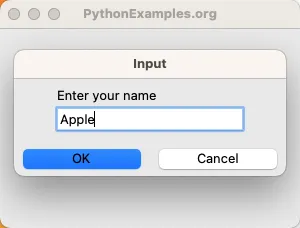
And clicks on OK button, then the entered value is returned by the askstring() method. The following greeting message would be displayed to the user.
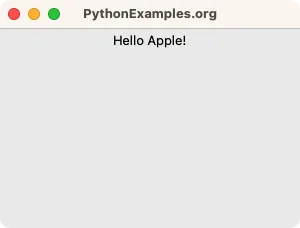
If user clicks on Cancel button, then askstring() returns None. And the following would be displayed in the main window.
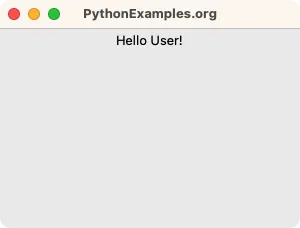
Summary
In this Python Tkinter tutorial, we learned how to prompt for an input from user in a dialog box in Tkinter, with examples.