Selenium Python – Check if checkbox is selected
In this tutorial, you will learn how to check if a checkbox in a form is selected (checked) or not, in Selenium Python.
To check if a checkbox is selected in Selenium Python, find the checkbox WebElement, and call is_selected() method of the checkbox object. The method returns true if the checkbox is selected, or false if not.
Examples
In this example, we shall consider loading the webpage at URL: https://pythonexamples.org/tmp/selenium/index-21.html. The contents of this webpage is given below. The webpage contains three checkboxes.
index.html
<html>
<body>
<h1>My Sample Form</h1>
<form id="myform">
<input type="checkbox" id="apple" name="apple" value="Apple">
<label for="apple"> I eat apples.</label><br>
<input type="checkbox" id="banana" name="banana" value="Banana" checked>
<label for="banana"> I eat bananas.</label><br>
<input type="checkbox" id="cherry" name="cherry" value="Cherry" checked>
<label for="cherry"> I eat cherries.</label><br>
</form>
</body>
</html>
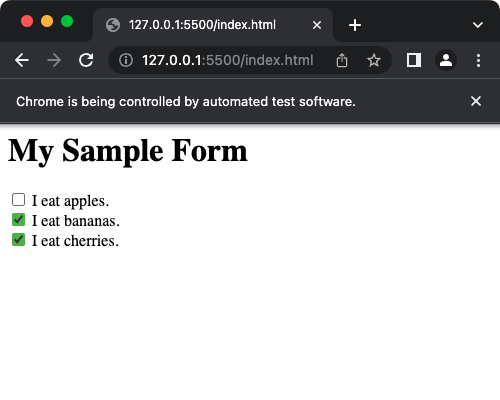
1. Example where Checkbox is selected
In this example, the checkbox we are interested is the one with the id="banana"
. And that checkbox is already checked via HTML script.
In the following program, we initialize a webdriver, navigate to the specified URL, get the checkbox with id attribute equal to "banana"
, and check if that checkbox is selected or not. We shall print a message to standard output if the checkbox is selected or not.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
import time
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(executable_path=ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-21.html')
# Find checkbox element
mycheckbox = driver.find_element(By.ID, 'banana')
# Check if checkbox is selected
if mycheckbox.is_selected():
print(f"{mycheckbox.get_attribute('value')} checkbox is selected.")
else:
print(f"{mycheckbox.get_attribute('value')} checkbox is not selected.")
# Wait to observe the window
time.sleep(5)
# Close the driver
driver.quit()
Output
Banana checkbox is selected.
2. Example where Checkbox is not selected
In this example, we shall load the webpage same as in the previous example, which contains three checkboxes. The checkbox we are interested in this example is the one with the id="apple"
. And that checkbox is not checked via HTML script.
In the following program, we initialize a webdriver, navigate to a specific URL (index.html) that is running on a local server, get the checkbox with id attribute value equal to "apple"
, and check if that checkbox is selected or not. We shall print a message to standard output if the checkbox is selected or not.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
import time
# Setup chrome driver
service = ChromeService(executable_path=ChromeDriverManager().install())
driver = webdriver.Chrome(service=service)
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-21.html')
# Find checkbox element
mycheckbox = driver.find_element(By.ID, 'apple')
# Check if checkbox is selected
if mycheckbox.is_selected():
print(f"{mycheckbox.get_attribute('value')} checkbox is selected.")
else:
print(f"{mycheckbox.get_attribute('value')} checkbox is not selected.")
# Wait to observe the window
time.sleep(5)
# Close the driver
driver.quit()
Output
Apple checkbox is not selected.
Summary
In this Python Selenium tutorial, we have given instructions on how to check if a checkbox is selected or not, using is_selected() method of the checkbox WebElement object.