Setup Selenium for Python
Setup Selenium
1. Install Selenium Libraries
To install Selenium libraries for Python programming, run the following command.
pip install selenium
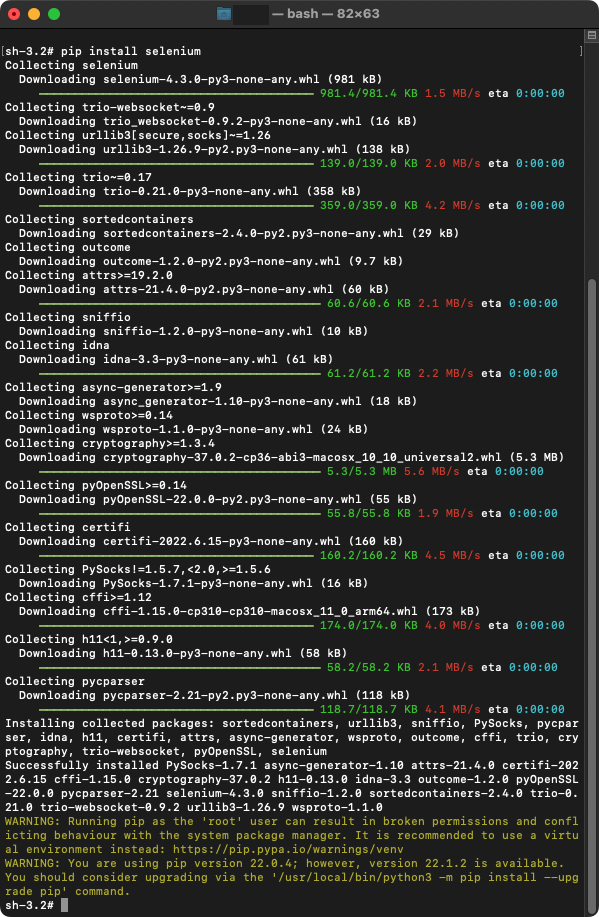
2. Download Browser Drivers
We can download browser drivers from the respective repositories. The links are given below.
Browser | Supported OS | Link |
---|---|---|
Chromium/Chrome | Windows/macOS/Linux | Downloads |
Firefox | Windows/macOS/Linux | Downloads |
Edge | Windows/macOS | Downloads |
Internet Explorer | Windows | Downloads |
Safari | macOS High Sierra and newer | Built in to Safari browser |
Download the browser driver based on the browser version in your PC/Mac.
For example, I would like to find out which driver I should download to use it my Mac for Chrome browser. So, I open Chrome browser and find out the version.
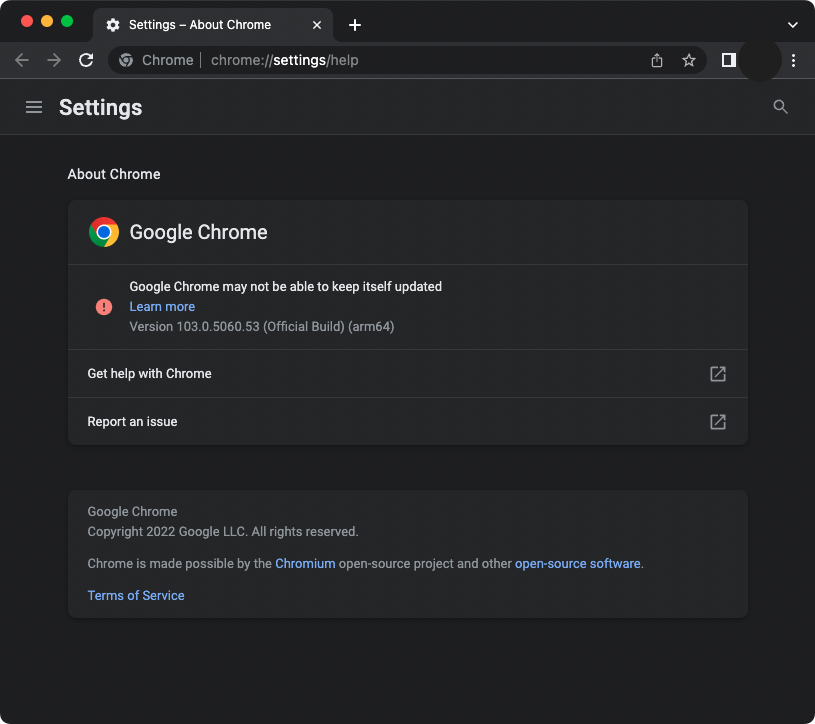
As you may have observed, the version is 103.0.5060.53. Therefore, we have to find this version in Chrome browser driver downloads page.
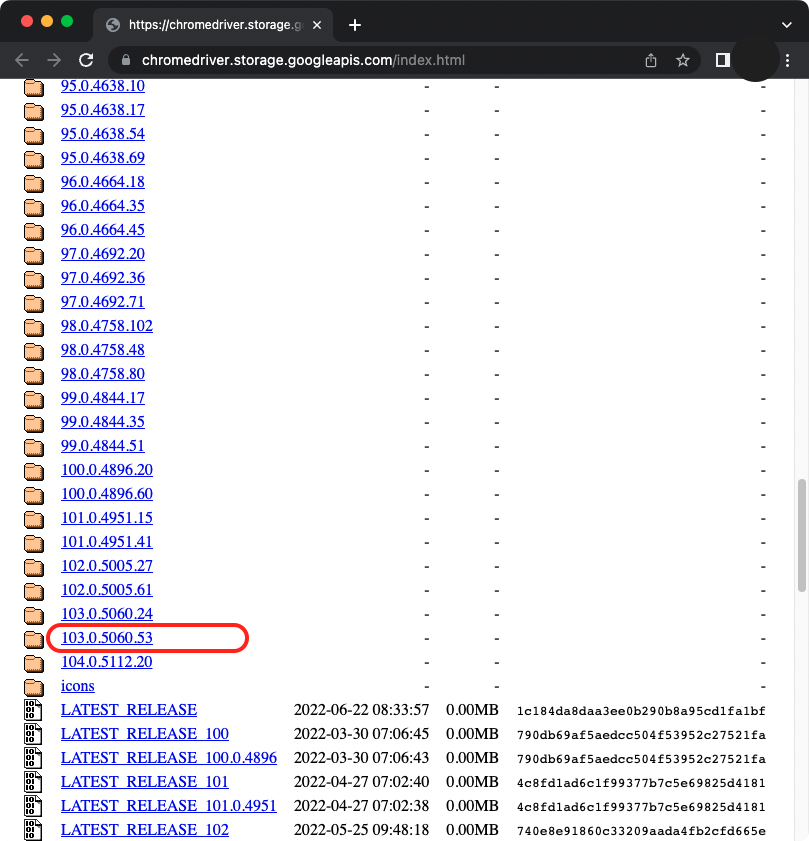
I am working on Mac, therefore, I download the chrome driver for Mac that is highlighted in the following screenshot.
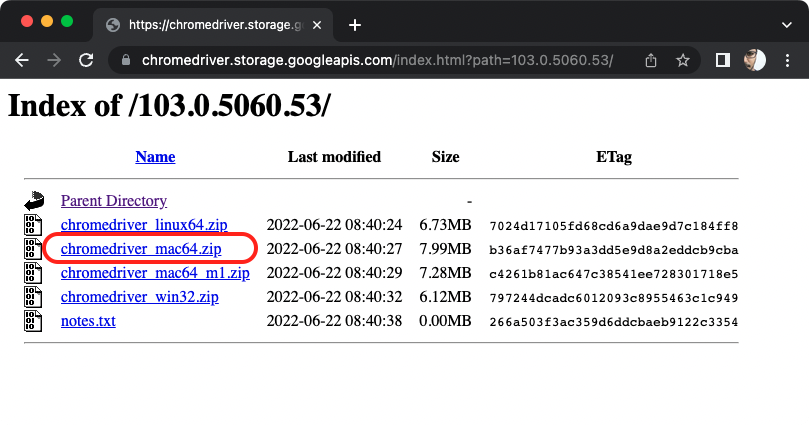
So, I download this driver that respects my Chrome version. You have to download the version that respects your browser and its version.
3. Make Driver Visible to Python Program
There are three ways in which we can make the driver visible to our Python code.
In this section, we will present two ways.
- Using PATH environment variable.
- Specifying hardcoded location in the Python program.
Once the respective driver is downloaded, you may add it the PATH based on your Operating System, or move it to a location and use that location hardcoded in the program.
I extracted the driver from zip file, and moved it the location /usr/local/bin/
.
Since our tutorials are for learning purpose, we specify hardcoded location in the Python program, as shown in the following.
from selenium.webdriver.chrome.service import Service
from selenium import webdriver
service = Service(executable_path="/usr/local/bin/chromedriver")
driver = webdriver.Chrome(service=service)
4. Selenium First Program
Now, we write our first program, to start Web Driver and open the url "https://www.google.com/"
.
Python Program
from selenium.webdriver.chrome.service import Service
from selenium import webdriver
service = Service(executable_path="/usr/local/bin/chromedriver")
with webdriver.Chrome(service=service) as driver:
driver.get('https://www.google.com/')
If you are working in Mac, and get a waring popup message that says "“chromedriver” cannot be opened because the developer cannot be verified.", you may execute the following command.
xattr -d com.apple.quarantine /usr/local/bin/chromedriver