How to get value in input text field in Selenium Python?
Selenium Python - Get value in input text field
In this tutorial, you will learn how to get or read the value entered by a user in an input text field in Selenium Python.
To get the value present in an input text field in Selenium Python, you can use get_attribute() method of the element object.
Steps to get the value present in input text field
- Find the input text field element in the web page using
driver.find_element()
method. - Call
get_attribute()
method on the input text field element, and pass the attribute name'value'
to the method. The method returns the value present in the input text field.
value = input_text_element.get_attribute('value')
Example
In the following example, we initialize a Chrome webdriver, navigate to a specific URL:/tmp/selenium/index-36.html, that contains a form element with a couple of input text fields, take the screenshot, get the value present in the input text field whose id="fname"
, and print the value to standard output.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 500)
# Navigate to the url
driver.get('/tmp/selenium/index-36.html')
# Take a screenshot of the webpage
driver.save_screenshot("screenshot.png")
# Find input text field
input_text_fname = driver.find_element(By.ID, 'fname')
# Get the value in the input text field
input_text_value = input_text_fname.get_attribute('value')
print(input_text_value)
# Close the driver
driver.quit()
Output
Hero
/tmp/selenium/index-36.html
<html>
<body>
<h3>My Form</h3>
<form action="">
<label for="fname">First name:</label>
<input type="text" id="fname" name="fname" value="Hero"><br><br>
<label for="lname">Last name:</label>
<input type="text" id="lname" name="lname" value="Super"><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
screenshot.png
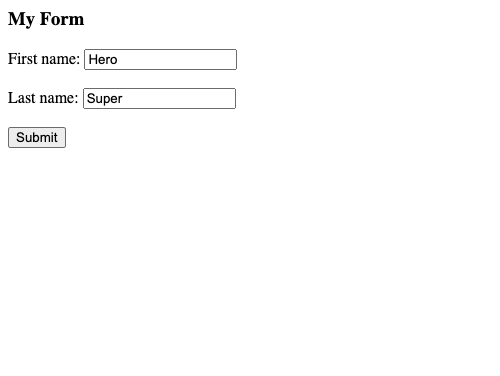
Summary
In this Python Selenium tutorial, we have given instructions on how to get the value in an input text field, using get_attribute() method.