Submit Form in Selenium
Selenium - Submit Form
To submit a form in Selenium Python, you can call the submit() method on the form element.
In this tutorial, you will learn how to find and submit a form in Selenium Python, with an example program.
Example
In this example, we shall consider loading the HTML file at path /tmp/selenium/index-35.html . The contents of this HTML file is given below.
<html>
<script>
function submitForm(){
console.log('form submitted.');
event.preventDefault();
}
</script>
<body>
<h1>My Sample Form</h1>
<form id="myform" onsubmit="someFunction()">
<label for="firstname">First name:</label>
<input type="text" id="myfirstname" name="firstname"><br><br>
<label for="lastname">Last name:</label>
<input type="text" id="mylastname" name="lastname"><br><br>
<label for="age">Age:</label>
<input type="text" id="myage" name="age"><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
In the following program, we initialize a Chrome webdriver, load URL for a webpage with a form element that has three input text fields. We shall find the form using id attribute, and then submit the form.
In the loaded webpage, upon form submission, submitForm() is called, which prints a message to console, and then prevents the default action of redirecting the page.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
import time
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 700)
# Navigate to the url
driver.get('/tmp/selenium/index-35.html')
# Find form element
myform = driver.find_element(By.ID, 'myform')
# Submit my form
myform.submit()
# Wait for 20s to observe console log
time.sleep(20)
# Close the driver
driver.quit()
Screenshot
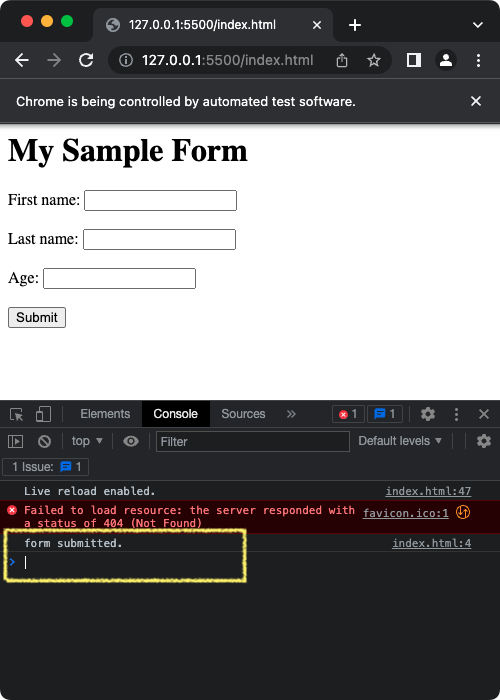
Summary
In this Python Selenium tutorial, we learned how to submit a form in the document, using submit() method, with the help of examples.