Get div text in Selenium
Selenium Python - Get Div Text
In this tutorial, you will learn how to get the text of a div element using Selenium in Python.
To get the text of a div element in Selenium, locate and get the div element, and then read the text parameter of this element. The WebElement.text returns the visible text of the div element.
The following code snippet returns the text of the div element mydiv
.
mydiv.text
Examples
In the following example, we shall consider loading the HTML file at path /tmp/selenium/index-1.html . The contents of this HTML file is given below.
<html>
<body>
<div id="mydiv1">Apple is red.</div>
<div id="mydiv2">Banana is yellow.</div>
<div id="mydiv3">Cherry is red.</div>
</body>
</html>
1. Get the text of div element whose id="mydiv2"
In the following program, we initialize a webdriver, navigate to a specific URL, get the div element whose id="mydiv2"
, get its text using text attribute, and print it to standard output.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
from selenium.common.exceptions import NoSuchElementException
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('/tmp/selenium/index-1.html')
# Get the div element by ID
try:
div_element = driver.find_element(By.ID, "mydiv2")
# Get text of div element
div_text = div_element.text
print(div_text)
except NoSuchElementException:
print("The div element does not exist.")
# Close the driver
driver.quit()
Output
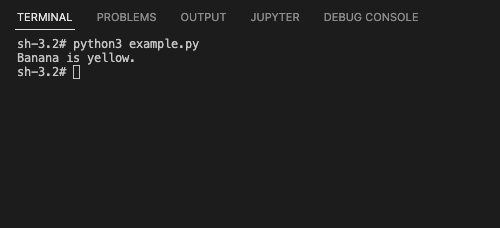
2. Get the text of all div elements
In the following program, we iterate over the div elements in the page, and print their text content.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('/tmp/selenium/index-1.html')
# Find all div elements
div_elements = driver.find_elements(By.TAG_NAME, "div")
for div_element in div_elements:
# Get text of div element
div_text = div_element.text
print(div_text)
# Close the driver
driver.quit()
Output
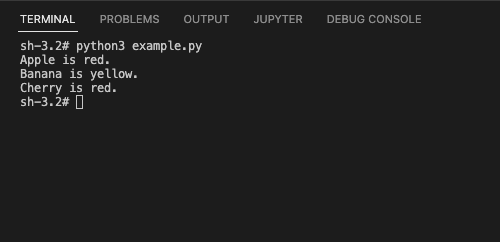
Summary
In this Python Selenium tutorial, we have given instructions on how to get the text content of div element using text
attribute of WebElement class, with example programs.