Contents
Selenium Python – Check if element is enabled
In this tutorial, you shall learn how to check if a given element in the page is enabled or not, using Selenium in Python.
If an element is enabled, then we can interact with it. Usually we check if an element is enabled or not, for form elements (like text input, checkboxes, and radio buttons). Because, if a form element is not enabled, it means that it is not currently interactable or cannot accept user input.
To check if an element is enabled in Selenium Python, find the element, and call the is_enabled() method on the element object. If the element is enabled, then the function returns True, else it returns False.
element.is_enabled()
We can use a Python if-else statement and the above expression as if-condition, as shown in the following code snippet.
if element.is_enabled():
print("The element is enabled.")
else:
print("The element is not enabled.")
Examples
1. Scenario where Element is enabled
In this example, we shall consider loading the HTML file at path https://pythonexamples.org/tmp/selenium/index-18.html . The contents of this HTML file is given below.
<html>
<body>
<h3>My Form</h3>
<form action="">
<label for="firstname">First name:</label>
<input type="text" id="firstname" name="firstname"><br><br>
<label for="lastname">Last name:</label>
<input type="text" id="lastname" name="lastname"><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
Copy 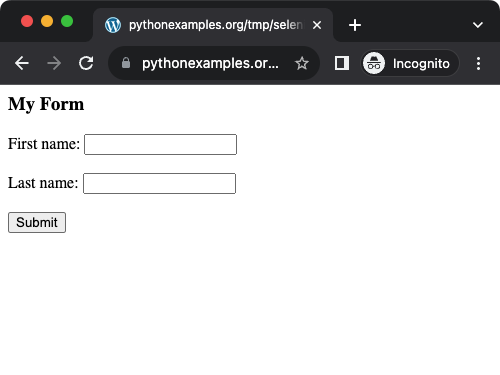
In the following program, we initialize a driver, load the specified URL, find the element with id="firstname", and check if the element is enabled or not using is_enabled() method of the web element object.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-18.html')
# Find the element by its ID
element = driver.find_element(By.ID, 'firstname')
# Check if the element is enabled
if element.is_enabled():
print("The element is enabled.")
else:
print("The element is not enabled.")
# Close the driver
driver.quit()
Output
The element is enabled.
2. Scenario where Element is not enabled
In this example, we shall consider loading the HTML file at path https://pythonexamples.org/tmp/selenium/index-34.html . The contents of this HTML file is given below.
<html>
<body>
<h3>My Form</h3>
<form action="">
<label for="firstname">First name:</label>
<input type="text" id="firstname" name="firstname" disabled><br><br>
<label for="lastname">Last name:</label>
<input type="text" id="lastname" name="lastname"><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
Copy 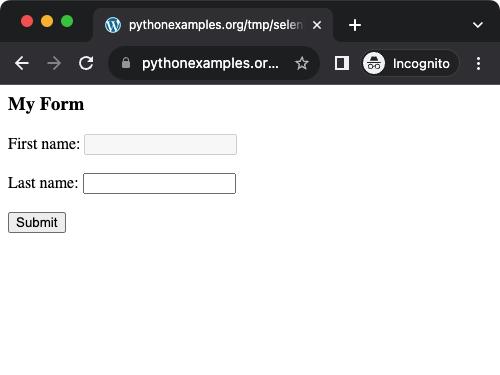
If you observe the HTML file, the element with id="firstname" has an attribute disabled. This disables the element from interaction. Now, we shall programmatically check if this element is enabled, using WebElement.is_enabled() method in Selenium.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-34.html')
# Find an element by its ID
element = driver.find_element(By.ID, 'firstname')
# Check if the element is enabled
if element.is_enabled():
print("The element is enabled.")
else:
print("The element is not enabled.")
# Close the driver
driver.quit()
CopyOutput
The element is not enabled.
Summary
In this Python Selenium tutorial, we have given instructions on how to check if given element is enabled or not, with example programs.