Selenium Python – Get Div inner HTML
In this tutorial, you will learn how to get the inner HTML of a div element using Selenium in Python.
To get the inner HTML of a div element in Selenium, locate and get the div element, call the get_attribute()
method on the div element and pass "innerHTML"
as argument. The method with the specified argument returns the inner HTML of the div element.
The following code snippet returns the inner HTML of the div element mydiv
.
mydiv.get_attribute("innerHTML")
Examples
In the following examples, we shall consider loading the HTML file at path https://pythonexamples.org/tmp/selenium/index-2.html . The contents of this HTML file is given below.
<html>
<body>
<div id="mydiv1">Apple is <span>red</span>.</div>
<div id="mydiv2">Banana is <span>yellow</span>.</div>
<div id="mydiv3">Cherry is <span>red</span>.</div>
</body>
</html>
1. Get the inner HTML of div element whose id=”mydiv2″
In the following program, we initialize a webdriver, navigate to a specific URL (index.html), get the div element whose id="mydiv2"
, get its inner HTML using get_attribute()
method, and print it to standard output.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
from selenium.common.exceptions import NoSuchElementException
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-2.html')
# Get the div element by ID
try:
div_element = driver.find_element(By.ID, "mydiv2")
# Get inner HTML of div element
div_inner_html = div_element.get_attribute("innerHTML")
print(div_inner_html)
except NoSuchElementException:
print("The div element does not exist.")
# Close the driver
driver.quit()
Output
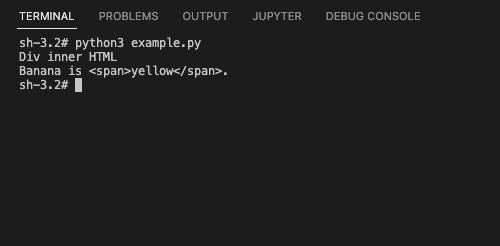
2. Get the inner HTML of all div elements
In the following program, we iterate over the div elements in the page, and print the inner HTML of each div.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-2.html')
# Find all div elements
div_elements = driver.find_elements(By.TAG_NAME, "div")
for index, div_element in enumerate(div_elements):
print(f"\nDiv {index + 1} inner HTML")
# Get inner HTML of div element
div_inner_html = div_element.get_attribute("innerHTML")
print(div_inner_html)
# Close the driver
driver.quit()
Output
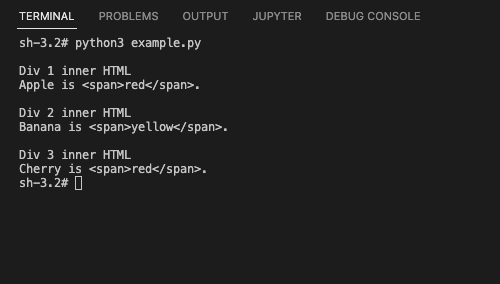
Summary
In this Python Selenium tutorial, we have given instructions on how to get the inner HTML of div element using get_attribute()
method of WebElement class, with example programs.