Get the parent element in Selenium
Selenium Python - Get the parent element
In this tutorial, you will learn how to get the parent element of a given element, in Selenium Python.
To get the parent element of a given element in Selenium Python, call the find_element() method on the given element and pass By.XPATH
for the by parameter, and '..'
for the value parameter in the function call.
If myelement
is the WebElement object for which we would like to find the parent, the code snippet for find_element() method is
myelement.find_element(By.XPATH, '..')
Example
In this example, we shall consider loading the webpage at URL: /tmp/selenium/index-19.html. The contents of this HTML file is given below.
The webpage contains a parent div with three children divs.
<html>
<body>
<h1>Hello Family</h1>
<div id="parent">
<div id="child1">This is child 1.</div>
<div id="child2">This is child 2.</div>
<div id="child3">This is child 3.</div>
</div>
</body>
</html>
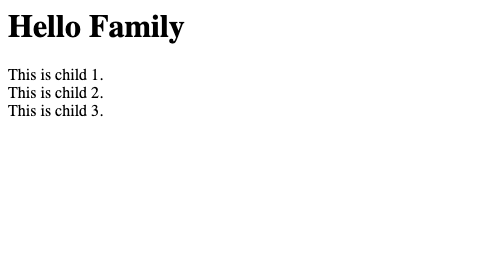
We shall take the first child div#child1
as our WebElement of interest, and find its parent div. We shall take the screenshot of the first child, and the parent.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service= ChromeService(executable_path=ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('/tmp/selenium/index-19.html')
# Get the div element you are interested in
mydiv = driver.find_element(By.ID, 'child1')
# Get parent of mydiv
parent = mydiv.find_element(By.XPATH, '..')
# Take sceenshots of mydiv, and its parent
mydiv.screenshot('screenshot-1.png')
parent.screenshot('screenshot-2.png')
# Close the driver
driver.quit()
Screenshot of child div element

Screenshot of parent div element

Summary
In this Python Selenium tutorial, we have given instructions on how to find the parent element of a given web element, with the help of an example program.