Selenium Python – Check if element exists
In this tutorial, you will learn how to check if an element with a specific locator exists in the webpage using Selenium in Python.
To check if an element exists in Selenium Python, find the element using a locator like type, id, or any attribute, or using XPath expression, with find_element()
method. If the method returns an element, then the element exists, but if the find_element()
method throws NoSuchElementException, then we can say that the element does not exist.
In the following code snippet, we use try-except to check if the element exists with the id='my-id-1'
.
try:
element = driver.find_element(By.ID, "my-id-1")
print("The element exists.")
except NoSuchElementException:
print("The element does not exist.")
Or if we use an XPath, then the code would be as shown in the following.
try:
element = driver.find_element(By.XPATH, "//*[@id='my-id-1']")
print("The element exists.")
except NoSuchElementException:
print("The element does not exist.")
Make sure that in the program you import NoSuchElementException from selenium.common.exceptions
.
Examples
In the following example, we shall consider loading the HTML file at path https://pythonexamples.org/tmp/selenium/index-11.html . The contents of this HTML file is given below.
index.html
<html>
<body>
<h2>Hello User!</h2>
<div>First div.</div>
<ul id="my-list-1">
<li>Apple</li>
<li>Banana</li>
<li>Cherry</li>
</div>
</body>
</html>
1. Scenario where the element exists
In the following program, we initialize a webdriver, navigate to a specific URL, and check if an element with id="my-list-1"
exists in the document or not.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
from selenium.common.exceptions import NoSuchElementException
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-11.html')
# Get the element
try:
element = driver.find_element(By.ID, "my-list-1")
print("The element exists.")
except NoSuchElementException:
print("The element does not exist.")
# Close the driver
driver.quit()
Output
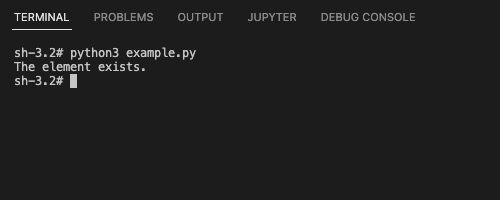
2. Scenario where the element does not exist
In this example, we are going to check if there is a paragraph in the document using By.TAGNAME
as strategy and "p"
as the locator for find_element()
method.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
from selenium.common.exceptions import NoSuchElementException
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-11.html')
# Get the element
try:
element = driver.find_element(By.TAG_NAME, "p")
print("The paragraph element exists.")
except NoSuchElementException:
print("The paragraph element does not exist.")
# Close the driver
driver.quit()
Output
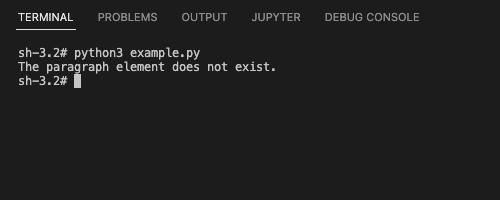
Summary
In this Python Selenium tutorial, we have given instructions on how to check if a specific element exists or not, with example programs.