Selenium Python – Get the child elements
In this tutorial, you will learn how to get the child elements of a given element, in Selenium Python.
To get the child elements of a given element in Selenium Python, call the find_elements() method on the given element and pass By.XPATH
for the by parameter, and '*'
for the value parameter in the function call.
If myelement
is the WebElement object for which we would like to find the child elements, the code snippet for find_elements() method is
myelement.find_elements(By.XPATH, '*')
The above method call returns a list of WebElement objects.
Example
In this example, we shall consider loading the webpage at URL: https://pythonexamples.org/tmp/selenium/index-19.html. The contents of this HTML file is given below. The web page contains a parent div with three children divs.
<html>
<body>
<h1>Hello Family</h1>
<div id="parent">
<div id="child1">This is child 1.</div>
<div id="child2">This is child 2.</div>
<div id="child3">This is child 3.</div>
</div>
</body>
</html>
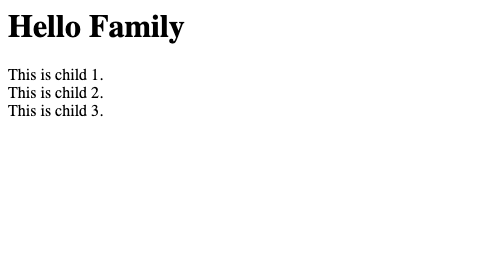
We shall take the parent div div#parent
as our WebElement of interest, find its child elements, and print these elements to standard output.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(executable_path=ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-19.html')
# Get the div element you are interested in
mydiv = driver.find_element(By.ID, 'parent')
# Get children of mydiv
children = mydiv.find_elements(By.XPATH, '*')
# Itertae over the children
for child in children:
print("\nChild Element")
print(child.get_attribute('outerHTML'))
# Close the driver
driver.quit()
Output
Child Element
<div id="child1">This is child 1.</div>
Child Element
<div id="child2">This is child 2.</div>
Child Element
<div id="child3">This is child 3.</div>
Summary
In this Python Selenium tutorial, we have given instructions on how to find the child elements of a given web element, with the help of an example program.