How to enter value in input text without send_keys() in Selenium Python?
Selenium Python - Enter value in input text field without send_keys()
In this tutorial, you will learn how to enter a value in an input text box without using send_keys() method in Selenium Python, but using execute_script() method.
To enter a value in an input text field in Selenium Python without using the send_keys() method, you can use execute_script() method of the driver object and execute a JavaScript code that sets value of the input text field.
Steps to enter value in input text field without send_keys()
- Find the input text box element in the web page using
driver.find_element()
method. - Call
execute_script()
method on the driver object, and pass the JavaScript code as argument. We can also pass addition arguments to theexecute_script()
method which in turn are passed to the JavaScript code.
value = "Apple"
driver.execute_script("arguments[0].value = '" + value + "'", input_field)
Example
In the following example, we initialize a Chrome webdriver, navigate to a specific URL that contains a form element with a couple of input text boxes, take the screenshot, enter a value in the input text field whose id is "fname"
using execute_script()
method, and then take the screenshot again.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 500)
# Navigate to the url
driver.get('/tmp/selenium/index-37.html')
# Find input text box
input_text_box_1 = driver.find_element(By.ID, 'fname')
# Take a screenshot before entering a value
driver.save_screenshot("screenshot-1.png")
# Enter a value in the input text box
value = "Apple"
driver.execute_script("arguments[0].value = '" + value + "'", input_text_box_1)
# Take a screenshot after entering a value
driver.save_screenshot("screenshot-2.png")
# Close the driver
driver.quit()
HTML File
The HTML file at URL: /tmp/selenium/index-37.html
<html>
<body>
<h1>My Form</h1>
<form action="" id="myform">
<label for="fname">First name:</label>
<input type="text" id="fname" name="fname"><br><br>
<label for="lname">Last name:</label>
<input type="text" id="lname" name="lname"><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
screenshot-1.png - Before setting the value in input text field
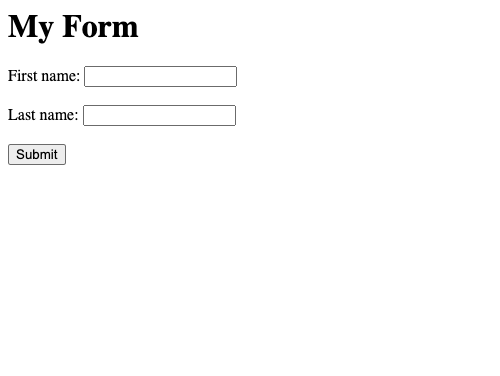
screenshot-2.png - After setting the value in input text field
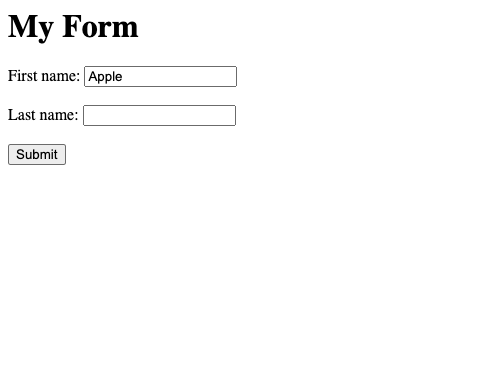
Summary
In this Python Selenium tutorial, we have given instructions on how to enter a value in an input text box without using send_keys() method. Instead, we used execute_script() method of the driver object.