Selenium Python – Get the next sibling element
In this tutorial, you will learn how to get the next sibling element of a given element, in Selenium Python.
To get the next or following sibling element of a given element in Selenium Python, call the find_element() method on the given element and pass By.XPATH
for by parameter, and 'following-sibling::*[1]
for the value parameter in the function call.
following-sibling::*
represents all the following sibling elements of a given element. But we need only the next one sibling element. Therefore we using array notation [1]
.
If myelement
is the WebElement object for which we would like to find the next sibling element, the code snippet for find_element() method is
myelement.find_element(By.XPATH, "following-sibling::*[1]")
The above method call returns a WebElement object containing the sibling element next to the given element.
Example
In this example, we shall consider loading the webpage at URL: https://pythonexamples.org/tmp/selenium/index-19.html. The contents of this HTML file is given below. The web page contains a parent div with three children divs.
<html>
<body>
<h1>Hello Family</h1>
<div id="parent">
<div id="child1">This is child 1.</div>
<div id="child2">This is child 2.</div>
<div id="child3">This is child 3.</div>
</div>
</body>
</html>
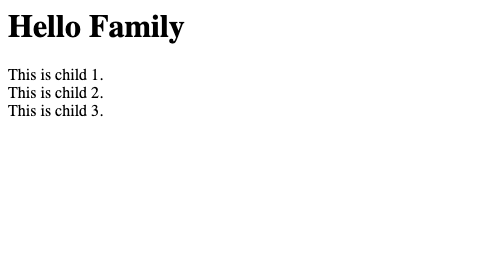
We shall take the child div div#child1
as our WebElement of interest, find its next sibling element, and print this element to standard output.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(executable_path=ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-19.html')
# Get the div element you are interested in
mydiv = driver.find_element(By.ID, 'child1')
# Get next sibling of mydiv
next_sibling = mydiv.find_element(By.XPATH, "following-sibling::*[1]")
print(next_sibling.get_attribute('outerHTML'))
# Close the driver
driver.quit()
Output
<div id="child2">This is child 2.</div>
Similarly,
- for child1: child2 is the next sibling element.
- for child2: child3 is the next sibling element.
- for child3, there is no next sibling element.
Summary
In this Python Selenium tutorial, we have given instructions on how to find the next sibling element of a given web element, with the help of an example program.