Selenium Python – Set window size
To set window size of the browser to a specific width and height in Selenium Python, you can use set_window_size() function of the webdriver object.
Call set_window_size()
function on the driver
object, and pass the width
(in pixels) and height
(in pixels) as arguments to the function.
width = 500
height = 400
driver.set_window_size(width, height)
In this tutorial, you will learn how to set the size of a browser window to a specific width and height in Selenium Python, with examples.
Examples
1. Set window size to 500×400
In the following example, we initialize a Chrome webdriver, and set its window size to a width of 500 pixels and a height of 400 pixels.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
import time
# Setup chrome driver
service = ChromeService(executable_path=ChromeDriverManager().install())
driver = webdriver.Chrome(service=service)
# Set window size
width = 500 # in pixels
height = 400 # in pixels
driver.set_window_size(width, height)
# Setting a delay so that we can see the browser window
time.sleep(5)
# Close the driver
driver.quit()
Browser window screenshot
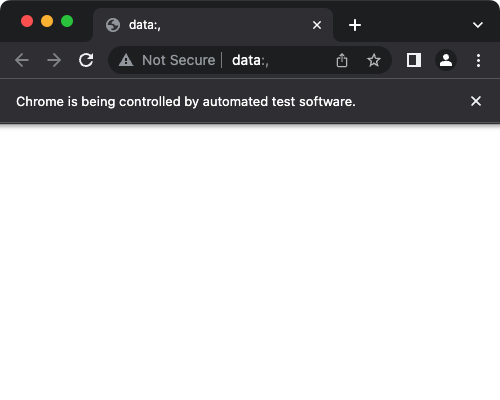
2. Set window size to 400×800
In the following example, we initialize a Chrome webdriver, and set its window size to a width of 400 pixels and a height of 800 pixels, something like a mobile view.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
import time
# Setup chrome driver
service = ChromeService(executable_path=ChromeDriverManager().install())
driver = webdriver.Chrome(service=service)
# Set window size
width = 400 # in pixels
height = 800 # in pixels
driver.set_window_size(width, height)
# Setting a delay so that we can see the browser window
time.sleep(5)
# Close the driver
driver.quit()
Browser window screenshot
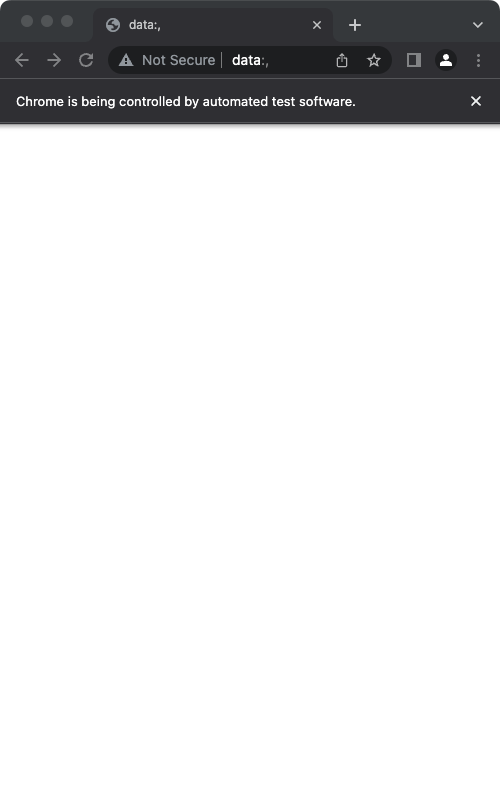
Summary
In this Python Selenium tutorial, we learned how to set the size of a browser window to specific width and height, using set_window_size() function.