Selenium Python – Uncheck the radio button
In this tutorial, you will learn how to unselect or uncheck a radio button in a group of related radio buttons using Selenium in Python.
To unselect a radio button in Selenium Python, find the radio button in the group which has been checked using XPath expression. Once you get the selected radio button, you can uncheck by running JavaScript on that element to set the checked property to false using execute_script()
method.
In the following code snippet, we get the selected radio button from a group with name="radio-group-name"
, and uncheck it using JavaScript.
radio_button = driver.find_element(By.XPATH, "//input[@type='radio' and @name='radio-group-name' and @checked]")
driver.execute_script("arguments[0].checked = false;", radio_button)
In the above code
- Selected
radio_button
is passed as second argument to execute_script(), which is then internally passed as argument to the JavaScript code. arguments[0]
gets the radio button.arguments[0].checked = false;
unchecks the radio button.
Example
In this example, we shall consider loading the webpage at URL: https://pythonexamples.org/tmp/selenium/index-17.html. The contents of this HTML file is given below.
<html>
<body>
<h3>My Favourite Subject</h3>
<form action="">
<input type="radio" id="physics" name="fav_subject" value="Physics">
<label for="physics">Physics</label><br>
<input type="radio" id="mathematics" name="fav_subject" value="Mathematics" checked>
<label for="mathematics">Mathematics</label><br>
<input type="radio" id="chemistry" name="fav_subject" value="Chemistry">
<label for="chemistry">Chemistry</label>
</form>
</body>
</html>
The webpage has a radio group with radio button value="Mathematics"
that has already been checked in the HTML using checked
attribute.
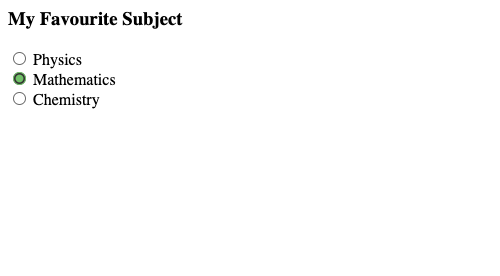
In the following program, we initialize a webdriver, navigate to a specified URL, and get the radio button with name equal to "fav_subject"
and attribute checked. We shall uncheck this radio button by running JavaScript via Selenium.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
import time
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-17.html')
# Get the selected radio button
radio_button = driver.find_element(By.XPATH, "//input[@type='radio' and @name='fav_subject' and @checked]")
time.sleep(2)
# Run custom JavaScript to uncheck the radio button
driver.execute_script("arguments[0].checked = false;", radio_button)
time.sleep(5)
# Close the driver
driver.quit()
time.sleep() statements are introduced to have a delay, so that we can see Selenium unchecking the radio button. Otherwise, it would be too fast, and we may think that the radio button is already not checked.
screenshot-1.png – Before unchecking the selected radio button.
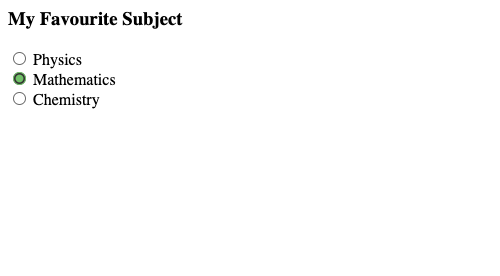
screenshot-2.png – After unchecking the selected radio button using Selenium.
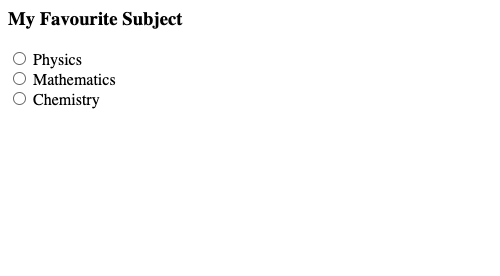
Summary
In this Python Selenium tutorial, we have given instructions on how to uncheck the selected radio button using Selenium via JavaScript, with an example.