Check if element has specific text using Selenium
Selenium Python - Check if element contains specific text
In this tutorial, you will learn how to check if an element has specific text using Selenium in Python.
To check if an element has specific text in Selenium Python, find the element using a locator with find_element()
method, and read the text attribute of the element. The text attribute returns a string containing the visible text of the element. Now, use Python-in keyword to check if this text content contains the specific text that we are searching.
Examples
In the following example, we shall consider loading the HTML file at path /tmp/selenium/index-9.html . The contents of this HTML file is given below.
<html>
<body>
<h2>Hello User!</h2>
<p id="msg" style="color:red;font-size:2rem;">This is my <strong>first</strong> paragraph. This is a sample text. Welcome!</p>
</body>
</html>
1. The element contains the search text
In the following program, we initialize a webdriver, navigate to a specific URL (index.html) that is running on a local server, and check if an element with id="msg"
contains the text "Welcome"
.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('/tmp/selenium/index-9.html')
# Get the element
element = driver.find_element(By.ID, "msg")
# Check if the element contains the text "Welcome"
search_text = "Welcome"
if search_text in element.text:
print(f"The element contains the {search_text}.")
else:
print(f"The element does not contain the search text: {search_text}.")
# Close the driver
driver.quit()
Output
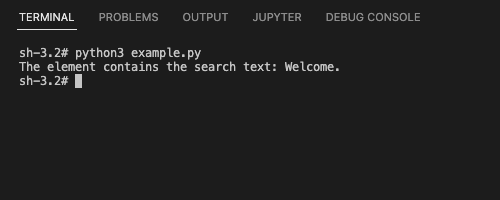
2. The element does not contain the search string.
In this example, we are going to search for the string "apple"
, in the paragraph with id="msg"
. We shall print in the output, whether the element text contains the given search string.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('/tmp/selenium/index-9.html')
# Get the element
element = driver.find_element(By.ID, "msg")
# Check if the element contains the text "apple"
search_text = "apple"
if search_text in element.text:
print(f"The element contains the search text: {search_text}.")
else:
print(f"The element does not contain the search text: {search_text}.")
# Close the driver
driver.quit()
Output
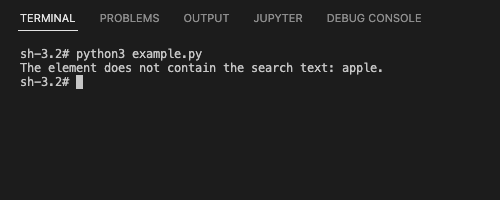
Summary
In this Python Selenium tutorial, we have given instructions on how to check if the text of element contains the given search string, with example programs.