Get text content of element in Selenium
Selenium Python - Get text content of an element
In this tutorial, you will learn how to get the text content of an element, using Selenium in Python.
To get the text content of an element, i.e., the visible text of an element in the webpage, in Selenium Python, locate the required element, and read the text attribute of the element object. The text attribute returns a string value representing the content in the element.
element.text
Examples
1. Get text content of an element
In this example, we shall consider loading the HTML file at path /tmp/selenium/index-13.html . The contents of this HTML file is given below.
index.html
<html>
<body>
<h2>Hello User!</h2>
<div id="parent">
<div id="child1">This is child 1.</div>
<div id="child2">This is child 2.</div>
<div id="child3">This is child 3.</div>
</div>
</body>
</html>
In the following program, we initialize a driver, then load the URL, find the element with id="child2"
, and get its text content using text attribute.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('/tmp/selenium/index-13.html')
# Find an element by its ID
element = driver.find_element(By.ID, 'child2')
# Get text content of element
text_content = element.text
print(text_content)
# Close the driver
driver.quit()
Output
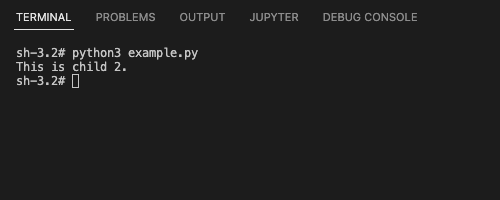
2. Get text content of an element with children
In the following program, we initialize a driver, then load the URL, the same one we used in the previous example. Find the element with id="parent"
, and get its text content using text attribute.
The element with id="parent"
has three children elements. The text of all the visible children is returned.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('/tmp/selenium/index-13.html')
# Find an element by its ID
element = driver.find_element(By.ID, 'parent')
# Get text content of element
text_content = element.text
print(text_content)
# Close the driver
driver.quit()
Output
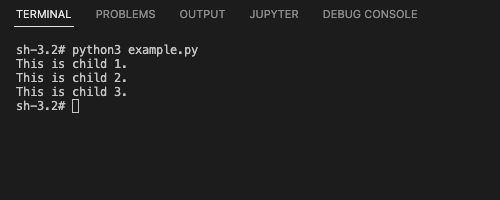
3. Get text content of an element with some of its children invisible
In this example, we shall consider loading the HTML file at path /tmp/selenium/index-14.html . The contents of this HTML file is given below.
<html>
<body>
<h2>Hello User!</h2>
<div id="parent">
<div id="child1">This is child 1.</div>
<div id="child2">This is child 2.</div>
<div id="child3" hidden>This is child 3.</div>
</div>
</body>
</html>
If we try to get the text content of div with id="parent"
, only the visible content is returned. Since the div with id="child3"
is hidden, the respective text is not returned.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('/tmp/selenium/index-14.html')
# Find an element by its ID
element = driver.find_element(By.ID, 'parent')
# Get text content of element
text_content = element.text
print(text_content)
# Close the driver
driver.quit()
Output
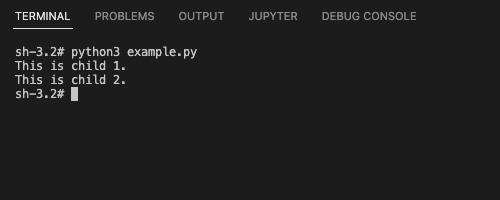
Summary
In this Python Selenium tutorial, we have given instructions on how to get the text content of an element, with example programs.