Enter value in input text box in Selenium
Selenium Python - Enter value in input text field
In this tutorial, you will learn how to enter a value in an input text field in Selenium Python.
To enter a value in an input text field in Selenium Python, you can call send_keys() method on the WebElement object, and pass the required value you want to input in the text field as argument.
Steps to enter value in input text field
- Find the input text field element in the web page using
driver.find_element()
method. - Call
send_keys()
method on the input text field element, and pass the value that we want to enter in the input text field, as argument.
input_text_element.send_keys("Ram")
Example
In the following example, we initialize a Chrome webdriver, navigate to a specific URL that contains a form element with a couple of input text fields, take the screenshot, enter a value in the input text field whose id is "fname"
, and then take the screenshot again.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 500)
# Navigate to the url
driver.get('/tmp/selenium/index-37.html')
# Find input text field
input_text_fname = driver.find_element(By.ID, 'fname')
# Take a screenshot before entering a value
driver.save_screenshot("screenshot-1.png")
# Enter a value in the input text field
input_text_fname.send_keys("Ram")
# Take a screenshot after entering a value
driver.save_screenshot("screenshot-2.png")
# Close the driver
driver.quit()
HTML File
The HTML file at URL: /tmp/selenium/index-37.html
<html>
<body>
<h3>My Form</h3>
<form action="">
<label for="fname">First name:</label>
<input type="text" id="fname" name="fname"><br><br>
<label for="lname">Last name:</label>
<input type="text" id="lname" name="lname"><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
screenshot-1.png
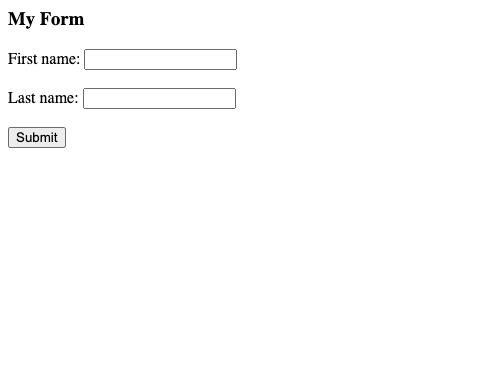
screenshot-2.png
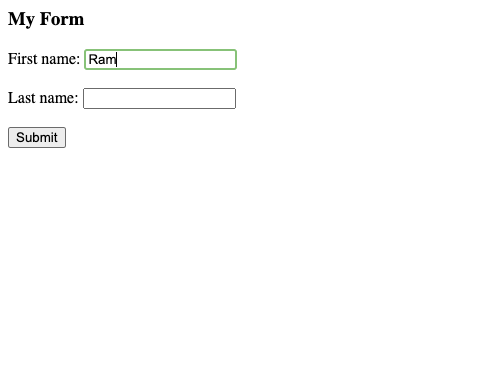
Summary
In this Python Selenium tutorial, we have given instructions on how to enter a value in an input text field, using send_keys() method.