Selenium – XPath for input text field
To find an input text field using XPath in Selenium, you can call the find_element() method on the driver object, with By.XPATH as the strategy (parameter), and required string value (that locates the input text field) for the locator parameter.
For example,
The following will return the first input text field present in the document.
driver.find_element(By.XPATH, '//input[@type="text"]')
The following will return the input text field with the specific id value present in the document.
driver.find_element(By.XPATH, '//input[@id="your-id-value"]')
//or
driver.find_element(By.XPATH, '//*[@id="your-id-value"]')
The following will return the input text field with the specific name value present in the document.
driver.find_element(By.XPATH, '//input[@name="your-name-value"]')
For any of the above expressions, you can include the type=”text” identifier in the XPath, as shown in the following.
driver.find_element(By.XPATH, '//input[@type="text" and @id="your-id-value"]')
driver.find_element(By.XPATH, '//*[@type="text" and @id="your-id-value"]')
driver.find_element(By.XPATH, '//input[@type="text" and @name="your-name-value"]')
Examples
In the following examples, we go through different scenarios of finding the input text field based on the identification attributes like name, id, or just the type, etc.
We shall use the following webpage at URL: https://pythonexamples.org/tmp/selenium/index-40.html, for the examples.
<html>
<body>
<h1>My Sample Form</h1>
<form action="" id="myform">
<label for="firstname">First name:</label>
<input type="text" id="myfirstname" name="firstname"><br><br>
<label for="lastname">Last name:</label>
<input type="text" id="mylastname" name="lastname"><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
1. Find first input text field using XPath
In the following program, we initialize a Chrome webdriver, load URL for a webpage with a form element that has two input text fields. We shall find the first input text field and set its value to "Apple"
.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-40.html')
# Find first input text field
my_input_text = driver.find_element(By.XPATH, '//input[@type="text"]')
# Enter a value in text field
my_input_text.send_keys("Apple")
# Take a screenshot after setting value
driver.save_screenshot("screenshot.png")
# Close the driver
driver.quit()
Screenshot
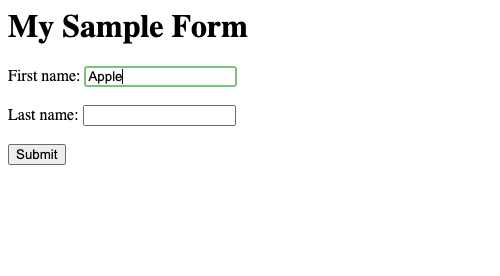
2. Find input text field using XPath based on name
In the following program, we find the input text element based on name attribute. We shall find the input text element whose name is "lname"
, and set its value to "Banana"
.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-40.html')
# Find input text field based on name attribute in XPath
my_input_text = driver.find_element(By.XPATH, '//input[@name="lastname"]')
# Enter a value in text field
my_input_text.send_keys("Banana")
# Take a screenshot after setting value
driver.save_screenshot("screenshot.png")
# Close the driver
driver.quit()
Screenshot
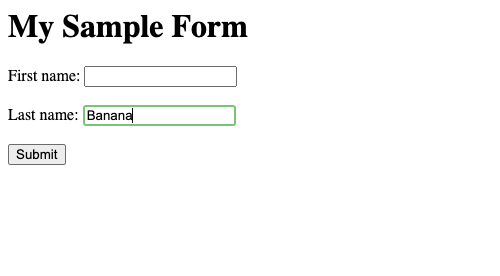
3. Find input text field using XPath based on id
In the following program, we find the input text element based on id attribute. We shall find the input text element whose id is "mylastname"
, and set its value to "Banana"
.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-40.html')
# Find input text field based on id attribute in XPath
my_input_text = driver.find_element(By.XPATH, '//input[@id="mylastname"]')
# Enter a value in text field
my_input_text.send_keys("Banana")
# Take a screenshot after setting value
driver.save_screenshot("screenshot.png")
# Close the driver
driver.quit()
Screenshot
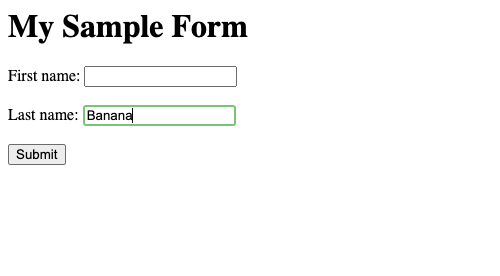
Summary
In this Python Selenium tutorial, we learned how to find a specific input text field in the document using XPath, with the help of examples.