Take screenshot of an element in Selenium
Selenium Python - Screenshot of an Element
In this tutorial, you will learn how to take the screenshot of a specific element in Selenium Python and save it to a specific path.
To take the screenshot of a single element in the webpage in Selenium Python, you can use screenshot() method of the element object.
Find the element, and call screenshot()
method on the element
object, and pass the location of the path as a string to which we would like to save the screenshot.
element.screenshot("path/to/screenshot.png")
Examples
In the following examples, we navigate to the following webpage at URL: /tmp/selenium/index-37.html, and take screenshots of the elements in it.
<html>
<body>
<h1>My Form</h1>
<form action="" id="myform">
<label for="fname">First name:</label>
<input type="text" id="fname" name="fname" value="Hero"><br><br>
<label for="lname">Last name:</label>
<input type="text" id="lname" name="lname" value="Super"><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
1. Take screenshot of a form element
In the following example, we initialize a Chrome webdriver, navigate to a specific URL, take the screenshot of the form with id 'myform'
, and save the screenshot to a location.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 500)
# Navigate to the url
driver.get('/tmp/selenium/index-37.html')
# Find form element
myform = driver.find_element(By.ID, 'myform')
# Take a screenshot of the form element
myform.screenshot("screenshot.png")
# Close the driver
driver.quit()
Screenshot
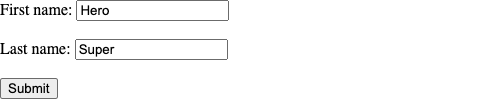
2. Take screenshot of a heading
In the following example, we initialize a Chrome webdriver, navigate to given URL, take the screenshot of the first heading h1
and save the screenshot as a PNG.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 500)
# Navigate to the url
driver.get('/tmp/selenium/index-37.html')
# Find form element
myform = driver.find_element(By.ID, 'myform')
# Take a screenshot of the form element
myform.screenshot("screenshot.png")
# Close the driver
driver.quit()
Screenshot

Summary
In this Python Selenium tutorial, we have given instructions on how to take the screenshot of a specific element in a webpage, and save it a to specific location, using screenshot() method of the element object.