Selenium Python – Get last paragraph
In this tutorial, you will learn how to get the last paragraph in the document using Selenium in Python.
In Selenium Python, call find_elements()
method of the WebDriver object and pass the arguments: By.TAGNAME
for by parameter and "p"
for the value parameter. The method returns all the paragraph elements as an array.
all_paragraphs = driver.find_elements(By.TAG_NAME, "p")
From this array you can get the last paragraph element in the document by getting the last element of the array using index=-1.
last_paragraph = all_paragraphs[-1]
Examples
1. Get the last paragraph in the document
In the following example, we shall consider loading the HTML file at path https://pythonexamples.org/tmp/selenium/index-5.html . The contents of this HTML file is given below.
<html>
<body>
<h2>Hello User!</h2>
<p>Apple is red.</p>
<p>Banana is yellow.</p>
<p>Cherry is red.</p>
</body>
</html>
In the following program, we initialize a webdriver, navigate to a specific URL, get the last paragraph element, and print the paragraph text to the standard output.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-5.html')
# Get the first paragraph element
all_paragraphs = driver.find_elements(By.TAG_NAME, "p")
last_paragraph = all_paragraphs[-1]
print(f"The last paragraph is :\n{last_paragraph.text}")
# Close the driver
driver.quit()
Output
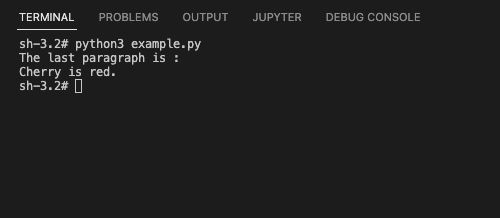
Summary
In this Python Selenium tutorial, we have given instructions on how to get the last paragraph element in the document using find_elements()
method of WebDriver class, with example programs.