Selenium Python – Select a radio button
In this tutorial, you will learn how to select a radio button in a form, in Selenium Python. We shall discuss two approaches of selecting of a radio button.
Approach 1
To select a radio button in Selenium Python, find the radio WebElement using appropriate selector such as an ID or XPath expression. Once you’ve located the radio button element, you can then call the click() method on the radio button element. This click operation on the radio button makes it selected.
In the following code snippet, we find the radio button whose name attribute is 'x', and value attribute is 'y', and select the radio button using click() method.
radio_button = driver.find_element(By.XPATH, "//input[@name='x' and @value='y']")
radio_button.click()
Now, let us see an example using click() method.
In this example, we shall consider loading the webpage at URL: https://pythonexamples.org/tmp/selenium/index-16.html. The contents of this HTML file is given below.
<html>
<body>
<h3>My Favourite Subject</h3>
<form action="">
<input type="radio" id="physics" name="fav_subject" value="Physics">
<label for="physics">Physics</label><br>
<input type="radio" id="mathematics" name="fav_subject" value="Mathematics">
<label for="mathematics">Mathematics</label><br>
<input type="radio" id="chemistry" name="fav_subject" value="Chemistry">
<label for="chemistry">Chemistry</label>
</form>
</body>
</html>
Copy In the following program, we initialize a webdriver, navigate to a specified URL, get the radio button with value equal to "Mathematics", and call click() method on this radio button element.
We shall take screenshots before and after selecting the radio button.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
service = ChromeService(executable_path=ChromeDriverManager().install())
driver = webdriver.Chrome(service=service)
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-16.html')
# Take a screenshot
driver.save_screenshot("screenshot-1.png")
# Get the radio element with value='Mathematics'
radio_button = driver.find_element(By.XPATH, "//input[@type='radio' and @value='Mathematics']")
# Click on the radio button element
radio_button.click()
# Take a screenshot
driver.save_screenshot("screenshot-2.png")
# Close the driver
driver.quit()
Copyscreenshot-1.png – Before selecting the Mathematics radio button
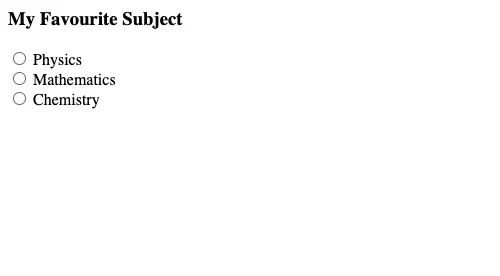
screenshot-2.png – After selecting the Mathematics radio button
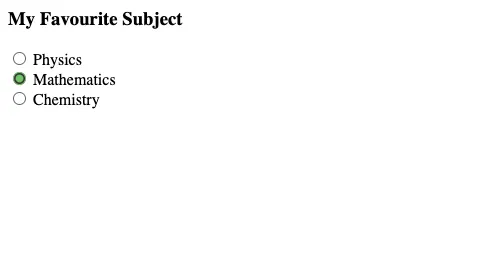
Approach 2
We can also use execute_script() method of driver object to select a radio button.
radio_button = driver.find_element(By.XPATH, "//input[@name='x' and @value='y']")
driver.execute_script("arguments[0].checked = true;", radio_button)
For the execute_script() method, we pass JavaScript code to set checked flag to true for the radio button element we pass in the second argument.
Let us consider the same URL from Approach 1 with three radio buttons. But we shall use execute_script() method of driver instead of click() method of web element, to select the radio button.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
service = ChromeService(executable_path=ChromeDriverManager().install())
driver = webdriver.Chrome(service=service)
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-16.html')
# Take a screenshot
driver.save_screenshot("screenshot-1.png")
# Get the radio element with value='Mathematics'
radio_button = driver.find_element(By.XPATH, "//input[@type='radio' and @value='Mathematics']")
# Set checked flag of radio button to true
driver.execute_script("arguments[0].checked = true;", radio_button)
# Take a screenshot
driver.save_screenshot("screenshot-2.png")
# Close the driver
driver.quit()
Copyscreenshot-1.png – Before selecting the Mathematics radio button
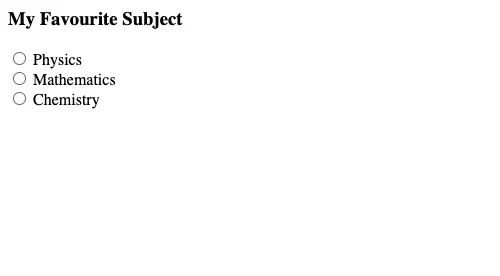
screenshot-2.png – After selecting the Mathematics radio button
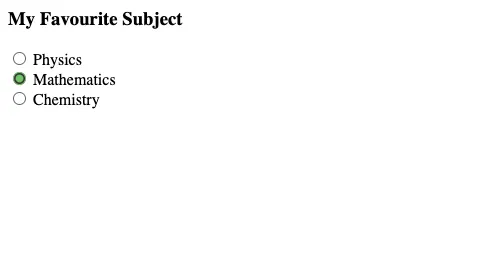
Summary
In this Python Selenium tutorial, we have given instructions on how to select a radio button using click() method of the checkbox WebElement object, or the execute_script() function of the driver element.