Selenium Python – Check if radio button exists
In this tutorial, you will learn how to check if a radio button exists in a webpage using Selenium in Python.
To check if a radio button exists in Selenium Python, find the radio button using a locator like type, id, or any attribute, or using XPath expression, with find_element()
method. If the method returns an element, then the radio button element exists, else if the method throws NoSuchElementException, then we can say that the radio button does not exist.
In the following code snippet, we use try-except to check if any radio button exists with the name='radio-group-name'
is
try:
radio_button = driver.find_element(By.XPATH, "//input[@type='radio' and @name='radio-group-name']")
print("Radio button exists.")
except NoSuchElementException:
print("Radio button does not exist.")
In the above XPath expression
//input
returns all the elements with input tag.@type='radio'
filters only those elements that are radio buttons.@name='radio-group-name'
filters only those radio buttons whose name attribute has a value equal to'radio-group-name'
.driver.find_element()
returns the first radio button from the filtered list, if it exists, else it raises NoSuchElementException.
Make sure that in the program you import NoSuchElementException from selenium.common.exceptions
.
Examples
1. Example where Radio button exists
In this example, we shall consider loading the HTML file at URL: https://pythonexamples.org/tmp/selenium/index-16.html. The contents of this HTML file is given below.
The webpage has a radio group for selecting your favorite subject with name="fav_subject"
.
index.html
<html>
<body>
<h3>My Favourite Subject</h3>
<form action="">
<input type="radio" id="physics" name="fav_subject" value="Physics">
<label for="physics">Physics</label><br>
<input type="radio" id="mathematics" name="fav_subject" value="Mathematics">
<label for="mathematics">Mathematics</label><br>
<input type="radio" id="chemistry" name="fav_subject" value="Chemistry">
<label for="chemistry">Chemistry</label>
</form>
</body>
</html>
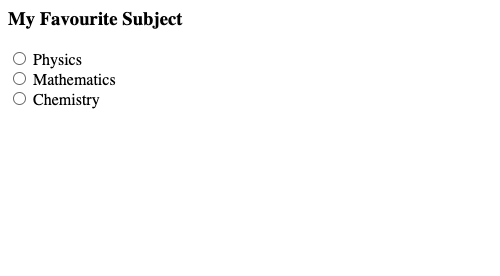
In the following program, we initialize a webdriver, navigate to a specified URL, and get the radio button whose name is equal to "fav_subject"
. We surround the code to get radio button in a try-except statement to check if a radio button exists or not. We shall print the result to standard output.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
from selenium.common.exceptions import NoSuchElementException
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-16.html')
# Get the radio button
try:
radio_button = driver.find_element(By.XPATH, "//input[@type='radio' and @name='fav_subject']")
print("Radio button exists.")
except NoSuchElementException:
print("Radio button does not exist.")
# Close the driver
driver.quit()
Output
Radio button exists.
2. Example where Radio button does not exist
In this example, we shall consider loading the HTML file at URL: https://pythonexamples.org/tmp/selenium/index-13.html. The contents of this HTML file is given below.
This webpage does not contain a radio button whose name is equal to "fav_subject"
, in fact it does not contain any radio button at all.
index.html
<html>
<body>
<h2>Hello User!</h2>
<div id="parent">
<div id="child1">This is child 1.</div>
<div id="child2">This is child 2.</div>
<div id="child3">This is child 3.</div>
</div>
</body>
</html>
Run the following program. Since there is no radio button with the specified selection criteria, except block would execute.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
from selenium.common.exceptions import NoSuchElementException
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-13.html')
# Get the radio button
try:
radio_button = driver.find_element(By.XPATH, "//input[@type='radio' and @name='fav_subject']")
print("Radio button exists.")
except NoSuchElementException:
print("Radio button does not exist.")
# Close the driver
driver.quit()
Output
Radio button does not exist.
Summary
In this Python Selenium tutorial, we have given instructions on how to check if a radio button exists or not, with example programs.