Selenium Python – Get the style attribute of an element
In this tutorial, you will learn how to get the style attribute of an element using Selenium in Python.
To get the style attribute of an element in Selenium Python, find the element, then call get_attribute()
method of the element object and pass "style"
as argument.
The get_attribute("style")
returns the style attribute of the element.
The following code snippet returns the inner HTML of the element
object.
element.get_attribute("style")
The element
is of type WebElement.
Examples
In the following example, we shall consider loading the HTML file at path https://pythonexamples.org/tmp/selenium/index-9.html . The contents of this HTML file is given below.
<html>
<body>
<h2>Hello User!</h2>
<p id="msg" style="color:red;font-size:2rem;">This is my <strong>first</strong> paragraph. This is a sample text. Welcome!</p>
</body>
</html>
1. Get the style of para element with id=”msg”
In the following program, we initialize a webdriver, navigate to a specific URL, get the style attribute of the element with id="msg"
, and print the style value to standard output.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-9.html')
# Get the element
element = driver.find_element(By.ID, "msg")
# Get style attribute of the element
style = element.get_attribute("style")
print(style)
# Close the driver
driver.quit()
Output
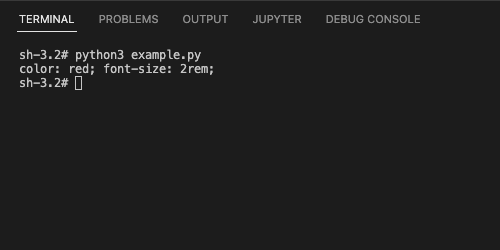
Summary
In this Python Selenium tutorial, we have given instructions on how to get the value of style attribute of an element using get_attribute()
method of WebElement class, with example programs.