Get all div elements in Selenium
Selenium Python - Get all div elements
In this tutorial, you will learn how to get all the div elements in the document using Selenium in Python.
To get all the div elements in Selenium Python, call find_elements()
method of the element object and pass the arguments: "By.TAGNAME"
for by parameter and "div"
for the value parameter.
The find_elements()
method returns a list of WebElement objects, where each object in the list is a div element.
The following code snippet returns all the div elements in the page.
driver.find_elements(By.TAG_NAME, "div")
Examples
1. Get all the "div" tag elements in the page
In the following examples, we shall consider loading the HTML file at path /tmp/selenium/index-4.html . The contents of this HTML file is given below.
<html>
<body>
<div id="mydiv1">Apple</div>
<div id="mydiv2">Banana</div>
<div id="mydiv3">Cherry</div>
</body>
</html>
In the following program, we initialize a webdriver, navigate to a specific URL, get all the div elements, and print them to standard output using a Python For loop statement.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('/tmp/selenium/index-4.html')
# Get all the div elements
div_elements = driver.find_elements(By.TAG_NAME, "div")
# Iterate over the div elements
for index, div in enumerate(div_elements):
print(f'Div Element {index+1}\n{div.text}\n')
# Close the driver
driver.quit()
Output
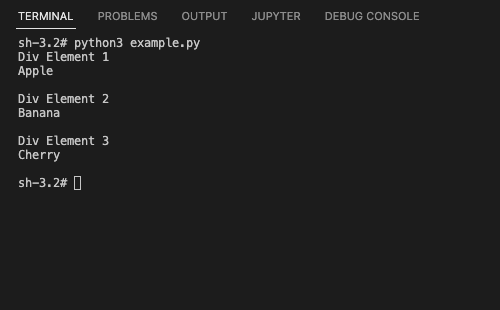
Summary
In this Python Selenium tutorial, we have given instructions on how to get all the div elements in the document using find_elements()
method of WebElement class, with example programs.