Selenium Python – Iterate over checkboxes
In this tutorial, you shall learn how to iterate over all the checkboxes in a webpage, in Selenium Python.
To iterate over all the checkboxes in Selenium Python, find all the checkboxes using find_elements() method of the driver object, and use a For loop or While loop to iterate over the checkboxes.
Example
In this example, we shall consider loading the webpage at URL: https://pythonexamples.org/tmp/selenium/index-22.html. The contents of this webpage is given below. The webpage contains three checkboxes.
index.html
<html>
<body>
<h1>My Sample Form</h1>
<form id="myform">
<input type="checkbox" id="apple" name="apple" value="Apple">
<label for="apple"> I eat apples.</label><br>
<input type="checkbox" id="banana" name="banana" value="Banana">
<label for="banana"> I eat bananas.</label><br>
<input type="checkbox" id="cherry" name="cherry" value="Cherry" checked>
<label for="cherry"> I eat cherries.</label><br>
</form>
</body>
</html>
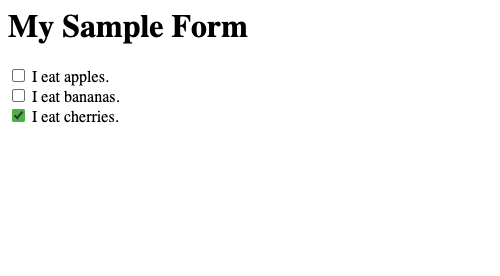
The first two checkboxes are not checked and the last checkbox is checked. We shall iterate over these checkboxes and print their information.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(executable_path=ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-22.html')
# Find all checkbox elements
checkboxes = driver.find_elements(By.XPATH, '//input[@type="checkbox"]')
# Iterate over checkboxes
for checkbox in checkboxes:
print(checkbox.get_attribute('value'))
print("is-selected : ", checkbox.is_selected())
print("name : ", checkbox.get_attribute('name'))
print()
# Close the driver
driver.quit()
Output
Apple
is-selected : False
name : apple
Banana
is-selected : False
name : banana
Cherry
is-selected : True
name : cherry
We can also access the index inside the For loop using enumerate() function. The code snippet to For loop with enumerate is given below.
# Iterate over checkboxes
for index, checkbox in enumerate(checkboxes):
print(f"Checkbox {index}" )
print("Value :", checkbox.get_attribute('value'))
print("is-selected : ", checkbox.is_selected())
print("name : ", checkbox.get_attribute('name'))
print()
Output
Checkbox 0
Value : Apple
is-selected : False
name : apple
Checkbox 1
Value : Banana
is-selected : False
name : banana
Checkbox 2
Value : Cherry
is-selected : True
name : cherry
Summary
In this Python Selenium tutorial, we have given instructions on how to iterate over all the checkboxes in a webpage using find_elements() method of the driver object with XPATH, and For loop statement.