Pressing Browser's Forward Button - Selenium
Pressing the Browser's Forward Button
To simulate pressing of the browser's forward button programmatically using Selenium for Python, call forward()
method on the Web Driver object.
driver.forward()
Example
- Initialise Chrome Web Driver object as
driver
. - Get the URL
/
using webdriver
object. - Find element by link text
'OpenCV'
and click on the element. - Press browser's back button using
driver.back()
. - Press browser's forward button using
driver.forward()
.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
import time
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(600,800)
# Navigate to the url
driver.get('/')
# Optional
time.sleep(5)
# Navigate to the url
driver.get('/selenium/')
# Optional
time.sleep(5)
# Simulate browser's back button
driver.back()
# Optional
time.sleep(5)
# Simulate browser's forward button
driver.forward()
# Optional
time.sleep(5)
# Close the driver
driver.quit()
Screenshots
Navigation Step #1 : Get the URL /
.
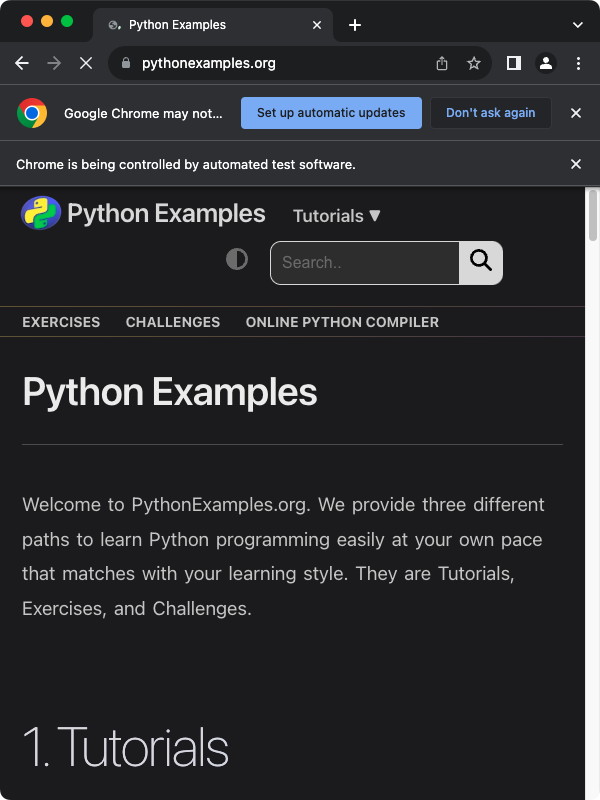
Navigation Step #2 : Navigate to Selenium tutorials page.
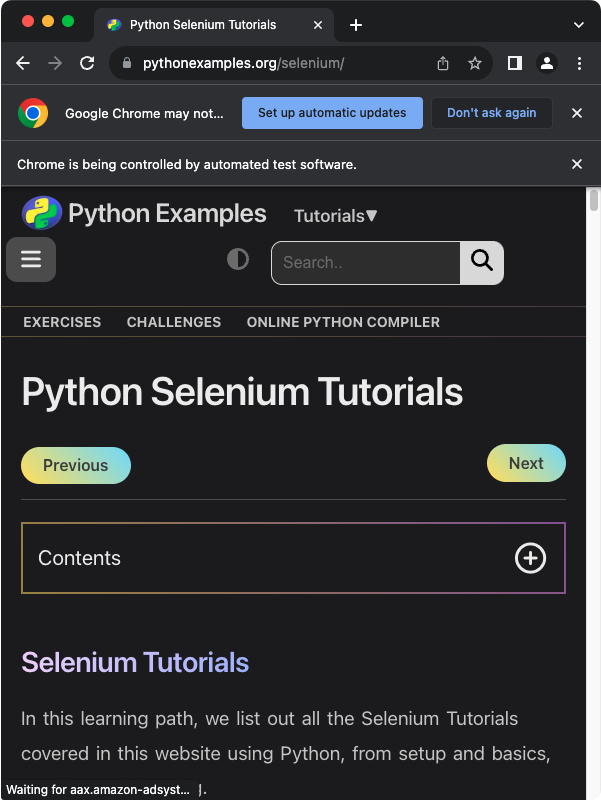
Navigation Step #3 : Press the browser's back button.
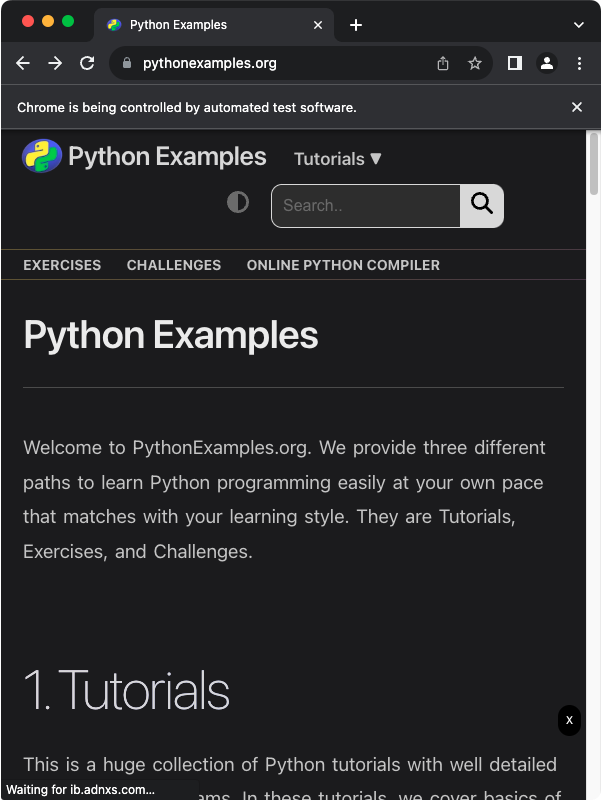
Navigation Step #4 : Press the browser's forward button.
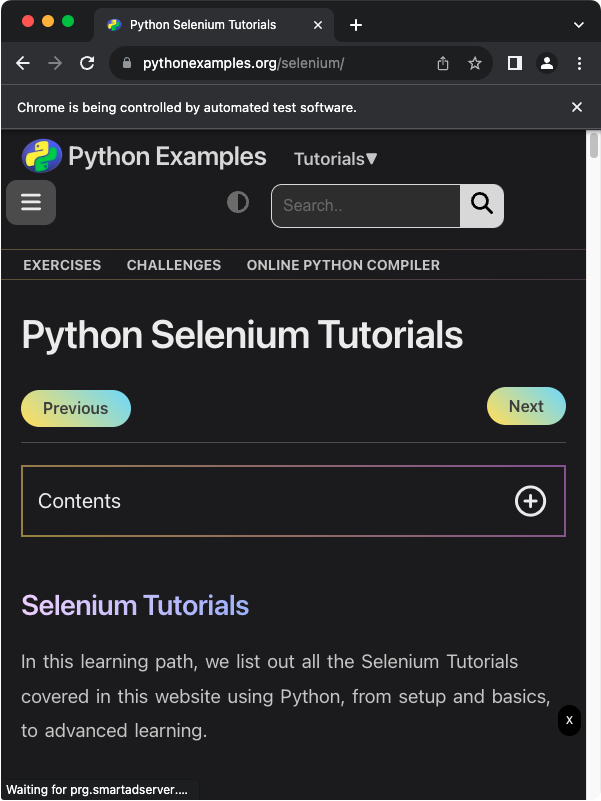
Summary
In this Python Selenium Tutorial, we learned how to press the browser's forward button using Selenium's driver.forward()
method.