Selenium Python – Unselect all checkboxes
In this tutorial, you will learn how to unselect all the checkboxes in a webpage, in Selenium Python.
To uncheck or unselect all the checkboxes in Selenium Python, find all the checkboxes using find_elements() method of the driver object, use a For loop or While loop to iterate over the checkboxes, and for each checkbox, check if the checkbox is selected or not, and if the checkbox is selected, then unselect it by calling click() function on the checkbox WebElement object.
Example
In this example, we shall consider loading the webpage at URL: https://pythonexamples.org/tmp/selenium/index-23.html. The contents of this webpage is given below. The webpage contains three checkboxes.
<html>
<body>
<h1>My Sample Form</h1>
<form id="myform">
<input type="checkbox" id="apple" name="apple" value="Apple">
<label for="apple"> I eat apples.</label><br>
<input type="checkbox" id="banana" name="banana" value="Banana" checked>
<label for="banana"> I eat bananas.</label><br>
<input type="checkbox" id="cherry" name="cherry" value="Cherry" checked>
<label for="cherry"> I eat cherries.</label><br>
</form>
</body>
</html>
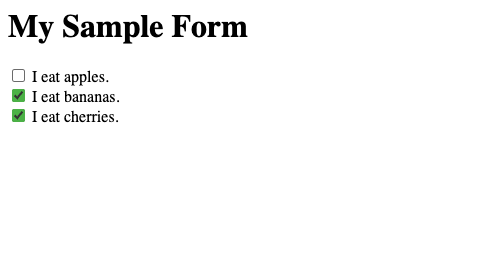
The first checkbox is not checked and the next two checkboxes are checked. We shall iterate over these checkboxes and unselect them all.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(executable_path=ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-23.html')
# Take a screenshot
driver.save_screenshot("screenshot-1.png")
# Find all checkbox elements
checkboxes = driver.find_elements(By.XPATH, '//input[@type="checkbox"]')
# Iterate over checkboxes
for checkbox in checkboxes:
# Unselect checkbox if already selected
if checkbox.is_selected():
checkbox.click()
print(f"{checkbox.get_attribute('value')} checkbox is unselected.")
else:
print(f"{checkbox.get_attribute('value')} checkbox is already unselected.")
# Take a screenshot
driver.save_screenshot("screenshot-2.png")
# Close the driver
driver.quit()
Output
Apple checkbox is already unselected.
Banana checkbox is unselected.
Cherry checkbox is unselected.
screenshot-1.png – Before unselecting all the checkboxes
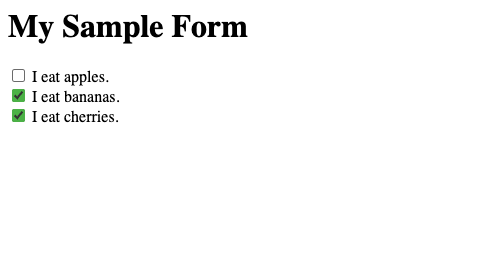
screenshot-2.png – After unselecting all the checkboxes
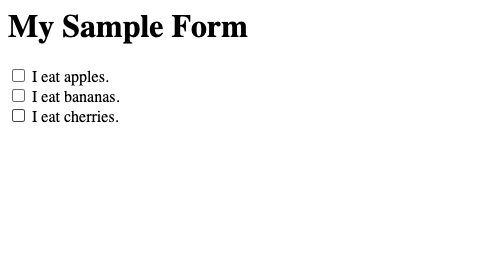
Summary
In this Python Selenium tutorial, we have given instructions on how to unselect all the checkboxes in a webpage, with the help of an example program.