Execute custom JavaScript code in Selenium
Selenium Python - Execute custom JavaScript code on Webpage
To execute a custom JavaScript code in a webpage in Selenium Python, you can use execute_script() method of the driver object.
Call execute_script()
method on the driver object, and pass the JavaScript code as argument. We can also pass additional arguments to the JavaScript code via execute_script()
method.
In this tutorial, you will learn how to execute a custom JavaScript code on the webpage, using execute_script() method in Selenium Python, with examples.
Examples
1. Execute JavaScript code, with no arguments
In the following example, we initialize a Chrome webdriver, navigate to a specific URL, and execute a JavaScript code to append text to the webpage, using execute_script()
method.
We shall take screenshots of the webpage before and after the JavaScript execution.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('/tmp/selenium/index-38.html')
# Take a screenshot before JS code execution
driver.save_screenshot("screenshot-1.png")
# Execute custom JavaScript
driver.execute_script("document.body.append('Apple Banana');")
# Take a screenshot after JS code execution
driver.save_screenshot("screenshot-2.png")
# Close the driver
driver.quit()
/tmp/selenium/index-38.html
<html>
<body>
<h1>Hello World</h1>
<p>This is a sample paragraph.</p>
</body>
</html>
screenshot-1.png - Before executing JavaScript
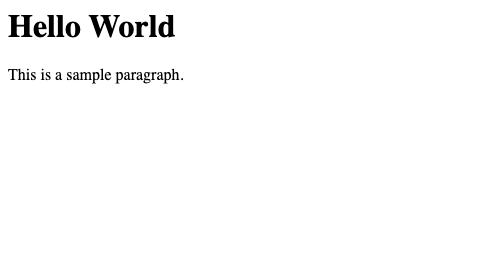
screenshot-2.png - After executing JavaScript
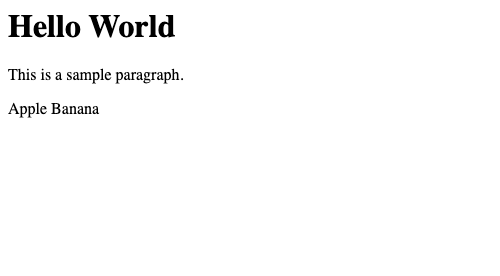
2. Pass arguments to the JavaScript code
In the following example, we pass additional arguments to the execute_script()
method, which are then passed as arguments
to the JavaScript code.
We shall pass the first heading h1
as argument to the JavaScript code, and change the color of h1
to 'blue'
in the JavaScript.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('/tmp/selenium/index-38.html')
# Take a screenshot before JS code execution
driver.save_screenshot("screenshot-1.png")
# Get first h1
h1 = driver.find_element(By.TAG_NAME, 'h1')
# Execute custom JavaScript
driver.execute_script("arguments[0].style.color='blue';", h1)
# Take a screenshot after JS code execution
driver.save_screenshot("screenshot-2.png")
# Close the driver
driver.quit()
screenshot-1.png - Before executing JavaScript
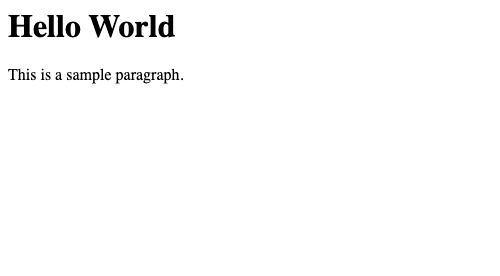
screenshot-2.png - After executing JavaScript
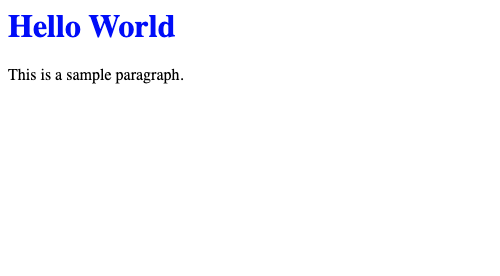
Summary
In this Python Selenium tutorial, we have given instructions on how to execute a custom JavaScript code on a webpage using Selenium Python, with examples.