Selenium Python – Check if element is visible
In this tutorial, you shall learn how to check if a given element in the page is visible or not, using Selenium in Python.
To check if an element is visible in Selenium Python, get the element using a By strategy and locator string, and call the is_displayed()
method on the element object. If the element is visible, then the function returns True, else it returns False.
element.is_displayed()
We can use a Python if-else statement and the above expression as if-condition, as shown in the following.
if element.is_displayed():
print("The element is visible.")
else:
print("The element is not visible.")
Examples
1. Scenario where Element is not visible
In this example, we shall consider loading the HTML file at path https://pythonexamples.org/tmp/selenium/index-14.html . The contents of this HTML file is given below.
<html>
<body>
<h2>Hello User!</h2>
<div id="parent">
<div id="child1">This is child 1.</div>
<div id="child2">This is child 2.</div>
<div id="child3" hidden>This is child 3.</div>
</div>
</body>
</html>
In the following program, we initialize a driver, load the specified URL, find the element with id="child3"
, and check if the element is visible or not using is_displayed()
method of the element object.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-14.html')
# Find an element by its ID
element = driver.find_element(By.ID, 'child3')
# Check if the element is visible
if element.is_displayed():
print("The element is visible.")
else:
print("The element is not visible.")
# Close the driver
driver.quit()
Output
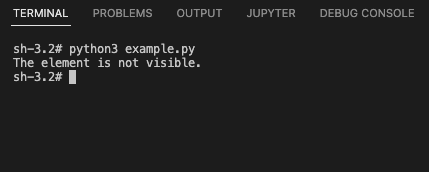
2. Scenario where Element is visible
In this example, we shall load the same HTML file as in the previous example. And we shall check if the element with id="child2"
is visible or not.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-14.html')
# Find an element by its ID
element = driver.find_element(By.ID, 'child2')
# check if the element is visible
if element.is_displayed():
print("The element is visible.")
else:
print("The element is not visible.")
# Close the driver
driver.quit()
Output
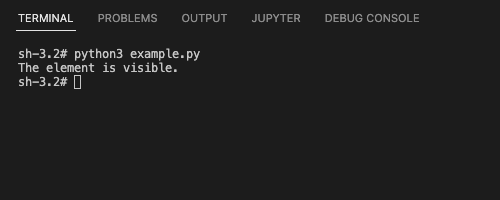
3. Scenario where Element is not visible by CSS
In this example, we shall consider loading the HTML file at path https://pythonexamples.org/tmp/selenium/index-15.html . The contents of this HTML file is given below.
The div with id="child1"
is hidden using CSS, meaning the div is not visible.
<html>
<head>
<style>
#child1 {
display: none;
}
</style>
</head>
<body>
<h2>Hello User!</h2>
<div id="parent">
<div id="child1">This is child 1.</div>
<div id="child2">This is child 2.</div>
<div id="child3" hidden>This is child 3.</div>
</div>
</body>
</html>
In the following program, we check if the element with id="child1"
is visible or not.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-15.html')
# Find an element by its ID
element = driver.find_element(By.ID, 'child1')
# check if the element is visible
if element.is_displayed():
print("The element is visible.")
else:
print("The element is not visible.")
# Close the driver
driver.quit()
Output
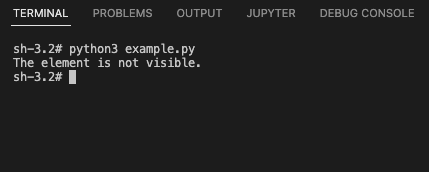
Summary
In this Python Selenium tutorial, we have given instructions on how to check if given element is visible or not in the webpage, with example programs.