Selenium Python – Select all checkboxes
In this tutorial, you will learn how to select all the checkboxes in a webpage, in Selenium Python.
To check or select all the checkboxes in Selenium Python, find all the checkboxes using find_elements() method of the driver object, use a For loop or While loop to iterate over the checkboxes, and for each checkbox, check if the checkbox is selected or not, and if the checkbox is not selected, then select by calling click() function on the checkbox WebElement object.
Example
In this example, we shall consider loading the webpage at URL: https://pythonexamples.org/tmp/selenium/index-22.html. The contents of this webpage is given below. The webpage contains three checkboxes.
<html>
<body>
<h1>My Sample Form</h1>
<form id="myform">
<input type="checkbox" id="apple" name="apple" value="Apple">
<label for="apple"> I eat apples.</label><br>
<input type="checkbox" id="banana" name="banana" value="Banana">
<label for="banana"> I eat bananas.</label><br>
<input type="checkbox" id="cherry" name="cherry" value="Cherry" checked>
<label for="cherry"> I eat cherries.</label><br>
</form>
</body>
</html>
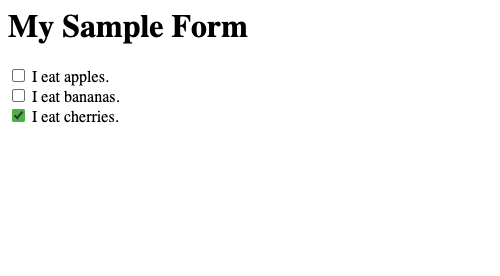
The first two checkboxes are not checked and the last checkbox is checked. We shall iterate over these checkboxes and select them all.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
from webdriver_manager.chrome import ChromeDriverManager
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(executable_path=ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-22.html')
# Take a screenshot
driver.save_screenshot("screenshot-1.png")
# Find all checkbox elements
checkboxes = driver.find_elements(By.XPATH, '//input[@type="checkbox"]')
# Iterate over checkboxes
for checkbox in checkboxes:
# Select checkbox if not already selected
if not checkbox.is_selected():
checkbox.click()
print(f"{checkbox.get_attribute('value')} checkbox is selected.")
else:
print(f"{checkbox.get_attribute('value')} checkbox is already selected.")
# Take a screenshot
driver.save_screenshot("screenshot-2.png")
# Close the driver
driver.quit()
Output
Apple checkbox is selected.
Banana checkbox is selected.
Cherry checkbox is already selected.
screenshot-1.png – Before selecting all the checkboxes
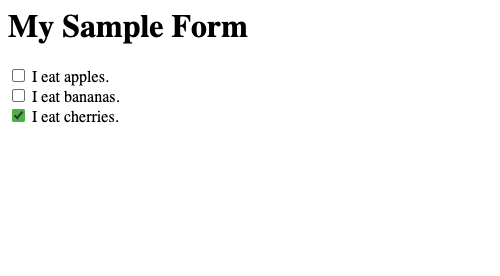
screenshot-2.png – After selecting all the checkboxes
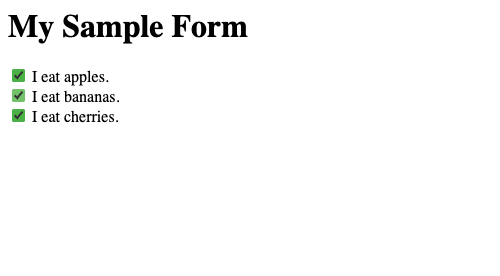
Summary
In this Python Selenium tutorial, we have given instructions on how to select all the checkboxes in a webpage, with the help of an example program.