Selenium Python – Select a checkbox
In this tutorial, you will learn how to select a checkbox in a form, in Selenium Python.
To select a checkbox in Selenium Python, find the checkbox WebElement, check if the checkbox is not selected, and if it is not selected, then call click() method of the checkbox object. This click operation on the checkbox makes the checkbox selected.
The method click()
makes a click on the checkbox element, and since we have already checked if the checkbox is not selected, the click action should make the checkbox selected.
Examples
In the following examples, we shall consider loading the webpage at URL: https://pythonexamples.org/tmp/selenium/index-22.html. The contents of this webpage is given below. The webpage contains three checkboxes, where the last checkbox is already checked via HTML script.
<html>
<body>
<h1>My Sample Form</h1>
<form id="myform">
<input type="checkbox" id="apple" name="apple" value="Apple">
<label for="apple"> I eat apples.</label><br>
<input type="checkbox" id="banana" name="banana" value="Banana">
<label for="banana"> I eat bananas.</label><br>
<input type="checkbox" id="cherry" name="cherry" value="Cherry" checked>
<label for="cherry"> I eat cherries.</label><br>
</form>
</body>
</html>
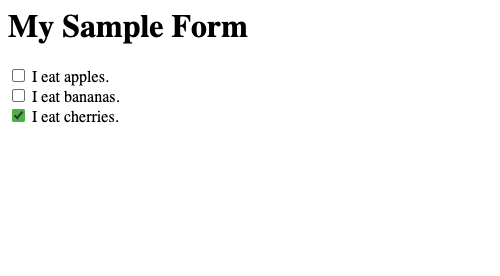
The first two checkboxes are not checked and the last checkbox is checked.
1. Select a Checkbox
In this example, the checkbox we are interested is the one with the id "cb1"
.
In the following program, we initialize a webdriver, navigate to the specified URL, get the checkbox with id attribute is equal to "apple"
, and call click()
method on this checkbox element.
We shall take screenshots before and after selecting the checkbox.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(executable_path=ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-22.html')
# Find checkbox element
mycheckbox = driver.find_element(By.ID, 'apple')
# Take a screenshot
driver.save_screenshot("screenshot-1.png")
# Select checkbox if not already selected
if not mycheckbox.is_selected():
mycheckbox.click()
print(f"{mycheckbox.get_attribute('value')} checkbox is selected.")
else:
print(f"{mycheckbox.get_attribute('value')} checkbox is already selected.")
# Take a screenshot
driver.save_screenshot("screenshot-2.png")
# Close the driver
driver.quit()
Output
Apple checkbox is selected.
screenshot-1.png – Before selecting the Apple checkbox
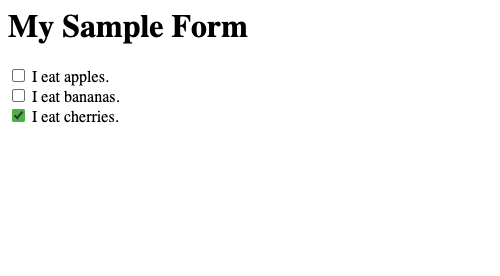
screenshot-2.png – After selecting the Apple checkbox
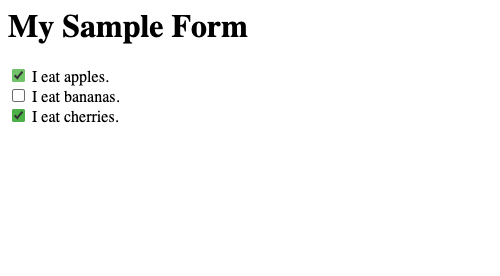
2. Select a Checkbox that is already selected
In this example, the checkbox we are interested is the one with the id "cb3"
which is already selected.
In the following program, we initialize a webdriver, navigate to the specified URL, get the checkbox with id attribute is equal to "cherry"
, and call click()
method on this checkbox element only if this checkbox is not already selected.
We are checking if the checkbox is selected using is_selected()
method of the checkbox object.
We shall take screenshots before and after selecting the checkbox.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(executable_path=ChromeDriverManager().install()))
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-22.html')
# Find checkbox element
mycheckbox = driver.find_element(By.ID, 'cherry')
# Take a screenshot
driver.save_screenshot("screenshot-1.png")
# Select checkbox if not already selected
if not mycheckbox.is_selected():
mycheckbox.click()
print(f"{mycheckbox.get_attribute('value')} checkbox is selected.")
else:
print(f"{mycheckbox.get_attribute('value')} checkbox is already selected.")
# Take a screenshot
driver.save_screenshot("screenshot-2.png")
# Close the driver
driver.quit()
Output
Cherry checkbox is already selected.
screenshot-1.png – Before selecting the Cherry checkbox
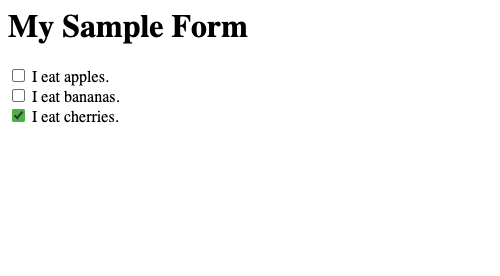
screenshot-2.png – After selecting the Cherry checkbox
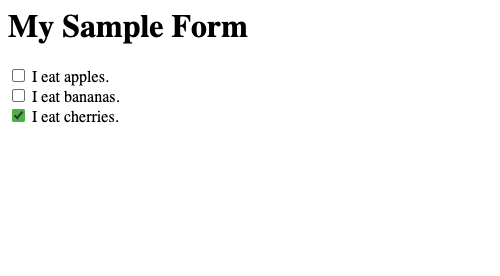
Summary
In this Python Selenium tutorial, we have given instructions on how to select a checkbox using click() method of the checkbox WebElement object.