Selenium Python – Select a random radio button
In this tutorial, you will learn how to select a random radio button using Selenium Python.
To select a random radio button using Selenium Python,
- Find the required group of radio buttons.
- Use random library to randomly pick a radio button from the group.
- Then use click() method on the radio button, to select it.
Example
In this example, we shall consider loading the webpage at URL: https://pythonexamples.org/tmp/selenium/index-16.html. The contents of this HTML file is given below.
The webpage has a radio group with three radio buttons.
<html>
<body>
<h3>My Favourite Subject</h3>
<form action="">
<input type="radio" id="physics" name="fav_subject" value="Physics">
<label for="physics">Physics</label><br>
<input type="radio" id="mathematics" name="fav_subject" value="Mathematics">
<label for="mathematics">Mathematics</label><br>
<input type="radio" id="chemistry" name="fav_subject" value="Chemistry">
<label for="chemistry">Chemistry</label>
</form>
</body>
</html>
Copy In the following program, we initialize a webdriver, navigate to a specified URL, get the radio buttons with name="fav_subject", and then randomly select one of them.
We shall take screenshots before and after selecting a random radio button.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
import random
# Setup chrome driver
service = ChromeService(executable_path=ChromeDriverManager().install())
driver = webdriver.Chrome(service=service)
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('https://pythonexamples.org/tmp/selenium/index-16.html')
# Take a screenshot
driver.save_screenshot("screenshot-1.png")
# Get the radio button whose name="fav_subject"
radio_buttons = driver.find_elements(By.XPATH, "//input[@type='radio' and @name='fav_subject']")
# Randomly pick a radio button
radio_button = random.choice(radio_buttons)
# Click the radio button
radio_button.click()
# Take a screenshot
driver.save_screenshot("screenshot-2.png")
# Close the driver
driver.quit()
Copyscreenshot-1.png – Before selecting a radio button
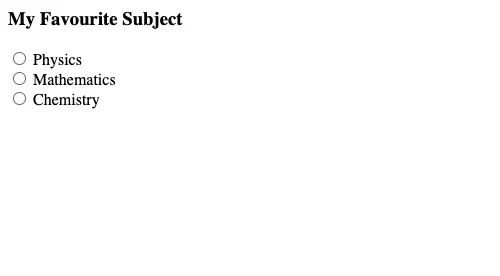
screenshot-2.png – After selecting a random radio button
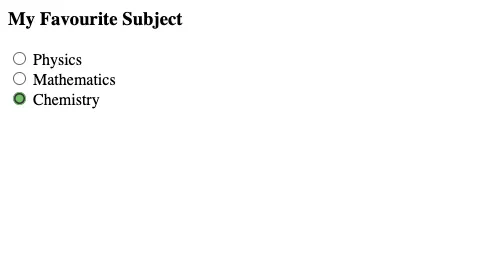
Summary
In this Python Selenium tutorial, we have given instructions on how to select a random radio button, with an example.