Check if element has id attribute set in Selenium
Selenium Python - Check if element has id
In this tutorial, you will learn how to check if an element has id attribute or not, using Selenium in Python.
To check if given web element has an id attribute or not, i.e., the id attribute of the element is set with a value or not, in Selenium Python, locate the required element, call the get_attribute()
function on the object with "id" passed as argument, and check if the returned value is an empty string.
If get_attribute()
returns an empty string, then the element has no id attribute, or else if the return value is not empty string, then the element has id attribute.
You can use an if-else conditional statement to check if the element has an id, as shown in the following.
if element.get_attribute('id') != "":
print("The element has an ID attribute.")
else:
print("The element does not have an ID attribute.")
Examples
1. Get text content of an element
In the following example, we shall consider loading the HTML file at path /tmp/selenium/index-12.html . The contents of this HTML file is given below.
<html>
<body>
<h2>Hello User!</h2>
<div id="parent">
<p id="child1">This is paragraph 1.</p>
<p>This is paragraph 2.</p>
</div>
</body>
</html>
In the following program, we initialize a driver, then load the specified URL, get all the paragraph elements, iterate over them and check if the paragraph element has id attribute or not.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('/tmp/selenium/index-12.html')
# Find an element by its ID
paras = driver.find_elements(By.TAG_NAME, 'p')
# Get text content of element
for para in paras:
print(para.text)
if para.get_attribute('id') != "":
print("The element has an ID attribute.")
else:
print("The element does not have an ID attribute.")
print()
# Close the driver
driver.quit()
Output
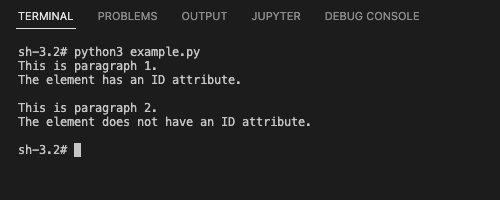
Summary
In this Python Selenium tutorial, we have given instructions on how to check if an element has an id attribute set or not, with example programs.