Get Div Elements with Specific Class Name in Selenium Python
Selenium Python - Get Div elements with specific class name
In this tutorial, you will learn how to get the div elements with a specific class name in the document using Selenium in Python, with examples.
To get the div elements with a specific class name in Selenium, call find_elements()
method on the driver object, and pass By.CLASS_NAME
for the by parameters, and your class name for the value parameter.
The following code snippet returns the all div elements with the class name "red"
.
driver.find_elements(By.CLASS_NAME, "red")
Examples
In the following examples, we shall consider loading the HTML file at path /tmp/selenium/index-1.html
. The contents of this HTML file is given below.
<html>
<body>
<div class="red">Apple is red.</div>
<div class="yellow">Banana is yellow.</div>
<div class="red">Cherry is red.</div>
</body>
</html>
1. Get all div elements with class name = "red"
In the following program, we initialize a webdriver, navigate to a specific URL, get all the div elements with class name = "red"
, and print them to output.
Python Program
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
# Setup chrome driver
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Navigate to the url
driver.get('/tmp/selenium/index-1.html')
# Find all div elements with specific class name
div_elements = driver.find_elements(By.CLASS_NAME, "red")
# Iterate over the divs
for div_element in div_elements:
print(div_element.text)
# Close the driver
driver.quit()
Output
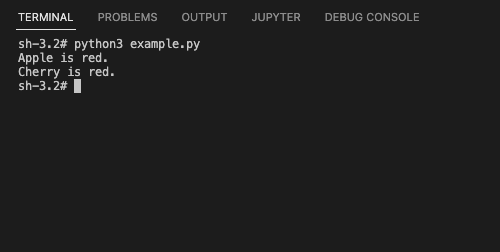
Summary
In this Python Selenium tutorial, we have given instructions on how to find all the div elements with a given class name using find_elements()
method of webdriver, with example programs.